Python is a popular programming language that is known for its simplicity, versatility, and ease of use. One important aspect of programming in Python is understanding the rules for naming variables.
While Python provides a lot of flexibility in how you can name your variables, there are some restrictions that you need to keep in mind.
One such restriction is that variables in Python cannot start with a number. In this article, we’ll explore why this rule exists, what happens if you break it, and how to follow best practices for naming variables in Python.
Whether you’re a beginner or an experienced Python developer, understanding these rules is essential for writing clean, readable, and maintainable code.
Back when I was a fresher and a noobie Python programmer, I could do some weird things that whenever I revisit code I had written before, I would feel “Uh c’mon Steve, you wrote that!”
As an example, just as I was learning about the basics of Python, I was introduced to variables. The hard part was not understanding how variables work, but how to piece the little piece and come up with a complete simple program.
I struggled! And I know you must too.
As I tried to write a program to calculate the average of set of two numbers, I would name my variables using weird names.
I could decide to name my variables based on the index of the number of the set. For example, the first number is named,
1
, the second number named2
, and so on.1 = 1 2 = 2 OR 1st_number = 1 2nd_number = 2
I did not know what I was doing.
But I would get the confidence to run the program.
And…
Hmmm!
I know, mate.
I would immediately encounter a syntax error and spend the rest of my day convincing myself I would never become a programmer. But, here I am! wiiii!
Eventually, I realized that Python naming conventions work a little bit different. Although, Python may have an English-like syntax, some rules do not apply here.
So, I decided to choose more meaningful and Python-like naming convention for my variables like
num1
andnumber2
. Eventually, I could get the confidence to run the program again, crossing my pinky finger, and voila, my code would run without any problem.My numbers would calculate successfully and I would spend the rest of the day convincing my friends to call me, master Steve! while bowing. Huh!
Through this experience, I learned the importance of choosing meaningful variable names and following the naming conventions for Python variables.
But also, learned that programming can be challenging at times, but with persistence and determination, I could overcome obstacles and achieve my goals.
So can Python variables start with a number?
Python variables cannot start with a number, as this is not a valid syntax for naming variables in Python. According to the official Python documentation, variable names must start with a letter or an underscore character, and can only contain letters, numbers, and underscores after that. If you attempt to use a variable name that starts with a number, you will encounter a syntax error in your code.
For example, the following variable names are valid in Python:
my_variable = 42
_my_variable = "hello"
myVariable = True
However, the following variable names are invalid because they start with a number:
2my_variable = 42 # invalid variable name
If you try to use an invalid variable name, Python will raise a SyntaxError
.
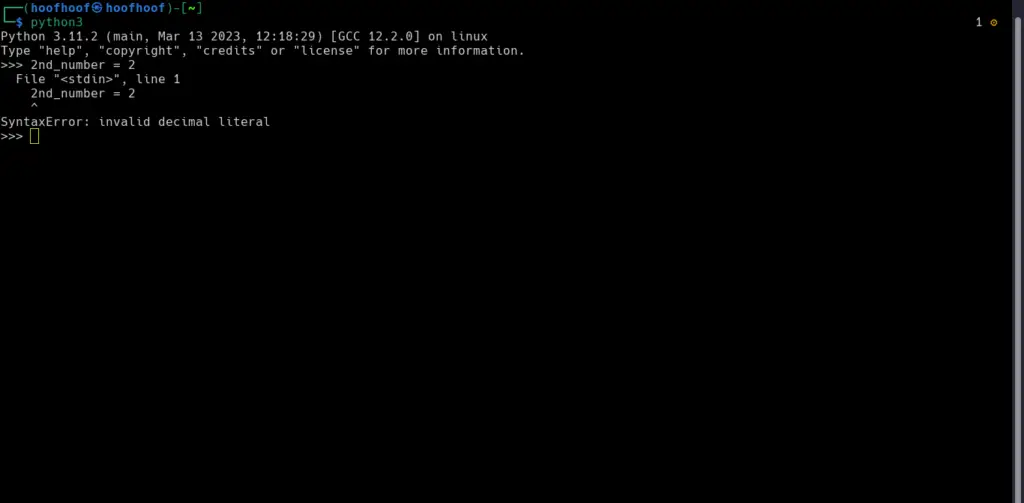
What is a variable name in Python?
A variable name is a label or term used to reference a reserved computer memory location, which in general terms, is called a variable. So, a variable name is a reference term for a variable.
There is a difference between a variable and a variable name.
A variable is a reserved computer memory location that is used to store values that the program may require as input for manipulation. These values can be integers, long integers, floats, and strings. Thus, a program requests this data stored in the memory location of the computer for processing or supporting the core operations of the Python program.
Variable names easily refer to memory addresses in a computer storing variables, i.e., integers, long integers, strings, and floats.
As a programmer, it would be so hard to reference the actual memory address in your Python code. An example of a memory location in hex format is 0x7f6835c88170.
So, instead of writing such a large hex number, programmers utilize variable names to label such a memory address in their code easily. For our hex example, I could easily reference the address location with a variable name x.
See the code below
x = 5
print(hex(id(x)))
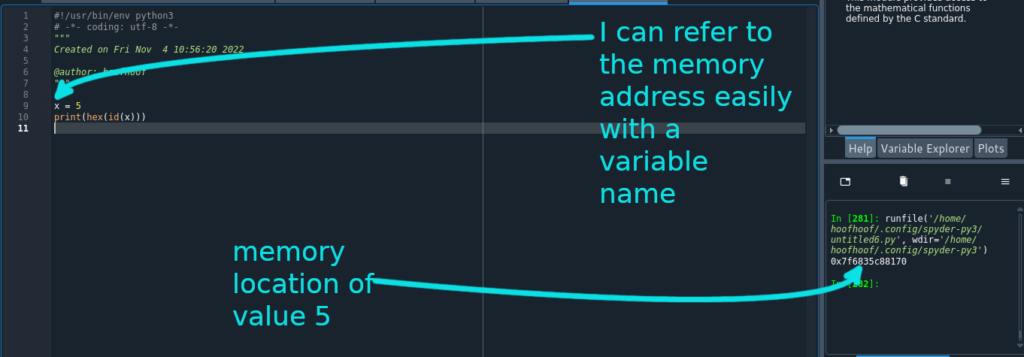
In Python, variable names are more than just names; they are labels that refer to specific objects in the program. When you declare a variable and assign it a value, you are essentially creating a label that points to a specific object in memory. For this reason, it’s important to follow the same rules for naming variables as you would for naming any other identifier in Python.
When naming variables, you should choose descriptive and meaningful names that accurately reflect the purpose of the variable.
This will make it easier for you and other developers to understand what the variable represents and how it is being used. You should also avoid using reserved keywords as variable names, as doing so can lead to unexpected behavior and errors in your code.
In summary, here are the most common rules of naming variables that you will most likely encounter:
- Python accepts only alphabets, underscores, and digits for naming variables. Variable names can only have letters in uppercase and lowercase, digits, and underscores.
- To name your variables, you can use alphanumeric characters (letters and digits) and underscores. Examples of valid variable names are article, my_var, var2, _var1, and my_var_with_underscores.
- You cannot use spaces in your variable names. Spaces are not permitted when naming your variables. Examples of invalid variable names are my var, var with spaces, var 1, etc.
- Variable names may not start with a digit. Thus, 1var, 2var, 3x, 2y, etc., are not valid for a variable name.
- The first letter should be a letter or an underscore.
- No two variables should have the same name. Each variable name must be unique in your Python program.
Now that you understand the rules of naming your variables let’s see how you can create a variable name in Python.
In Python, you declare a variable the moment you assign a value to it. To assign a value to a variable, you must use an equal sign to do that. Here is the syntax:
variable_name = value
my_var = 10
my_var is an example of a variable name that references the value 10 in the computer’s memory.
As you have noticed, you can join multiple words used in a variable name using underscores. So, if your descriptive name for a variable fits more than one word, use underscores to join them.
Another example of a variable using underscores is is_student, speed_limit, variable_y, e.t.c.
Types of data that variable can hold
- Integer, e.g., 1
- String, e.g., ‘Penelope‘
- Float, e.g., 3.45
- Long integer, e.g., a very long number with too many figures
Generally, you should not use special characters, single numbers, and non-descriptive texts to name your variables in Python.
Why can’t Python variables start with a number?
In Python, variables cannot start with a number because it is not a valid syntax for naming variables. Python variable names must start with a letter or an underscore character because Python uses the first character of a variable name to determine its type and scope.
Therefore, if you name your variables starting with a number, Python will automatically determine the data type of the variable type to be an integer. The variable being an integer, it can never be used as a variable name because another naming convention prohibits using integers during assignment (you cannot assign an integer with another interger in Python). (2 = 3 does not make sense)
Allowing variables to start with a number could lead to confusion because the interpreter might mistake the variable for a numeric literal.
For example, if you try to name a variable, 2my_variable
, the interpreter might interpret it as the number 2
followed by the variable name my_variable
. This would result in a syntax error besides making it difficult to read and understand the code.
FAQ
What kind of error will you get if you begin a variable with a number instead of a letter?
If you try to begin a variable with a number in Python, you will encounter a SyntaxError
with a message similar to the following: SyntaxError: invalid decimal literal
If you try to start a variable name with a digit in Python, you will also encounter a SyntaxError: invalid decimal literal
. This is because variable names in Python must follow certain naming conventions, and starting a variable name with a digit is not allowed.
For example, if you try to declare a variable as 2 = 3
, Python will raise a SyntaxError
with a message like the following:
2 = 3
^
SyntaxError: cannot assign to literal
In this case, the error occurred because 2
is not a valid variable name in Python. It is interpreted as a numeric literal, and you cannot assign a value to a literal in Python.
Conclusion
In conclusion, it’s important to follow Python’s variable naming conventions to write clean, readable, and maintainable code.
Variables should always be named in a way that accurately reflects their purpose, and they should be easy to understand by other developers.
While it may be tempting to try to start variable names with numbers or use other unconventional naming conventions, doing so will only lead to confusion and errors. By following best practices for naming variables in Python, you can improve the quality of your code, avoid syntax errors, and make it easier to collaborate with others by writing code that can be easily read and understood.