In other programming languages, such as JavaScript, it is common to use && operator to write logical statements that check if both items that are compared are the same.
Whether the uniqueness matches or not, the logical operator && returns a boolean value of True or False.
Logical operations are also evident in Python, and they pretty much form the basics of the language.
However, you will find another syntax used in Python to perform the logical-AND operations.
And if you’re asking whether you can use && in Python, then this article is for you.
So,
Can I use && operator in Python?
You cannot use && operator in Python to perform logical operations. Python will throw SyntaxError: invalid syntax error whenever you write && in your code. Instead, you should use && operator equivalent in Python AND to perform logical-and operations. Python uses AND instead of &&.
When you are getting started, you may be asking what is && in Python. && operator is used in some programming languages for logical operations.
However, in Python, && is not a valid syntax that can be used to do AND evaluation.
To show you, with an actual code, here is the error that Python throws whenever your try to use && operator :
if (2 == 2) && (3 == 3):
print("All numbers are equal")
My IDE already shows me an error when I try to use && in my code. The same happens when I execute my Python program:
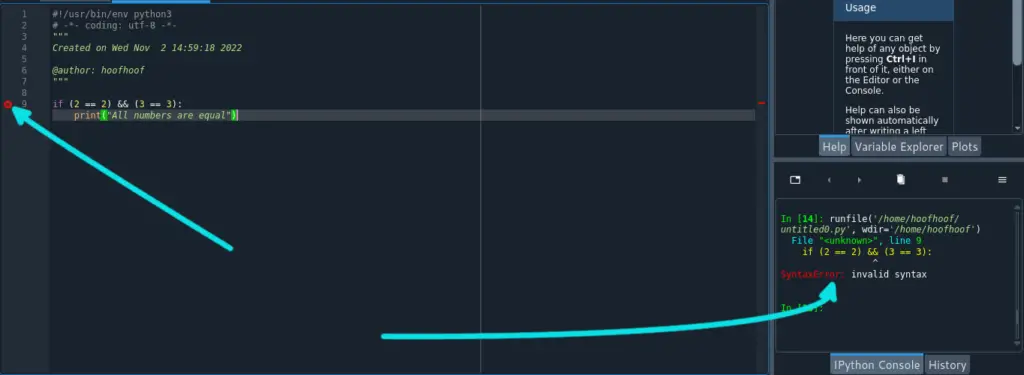
Instead, to write the correct logical AND operation using the correct syntax in Python, you must use the ‘and’ as the keyword.
The reason is that Python does not have && provisioned in either of its default and reserved keywords.
Thus, you are obliged to use AND instead of &&.
Here’s how to use Python’s equivalent of && in a typical if statement for comparison:
The code below shows how to use AND in an if statement in Python.
if (2 == 2) and (3 == 3):
print("All numbers are equal")
As I have used the correct syntax, my IDE shows me that I do not have any syntax errors in my code.
Besides, executing the program produces the results I want without throwing any syntax error as it previously did with the ‘&&’ operator.
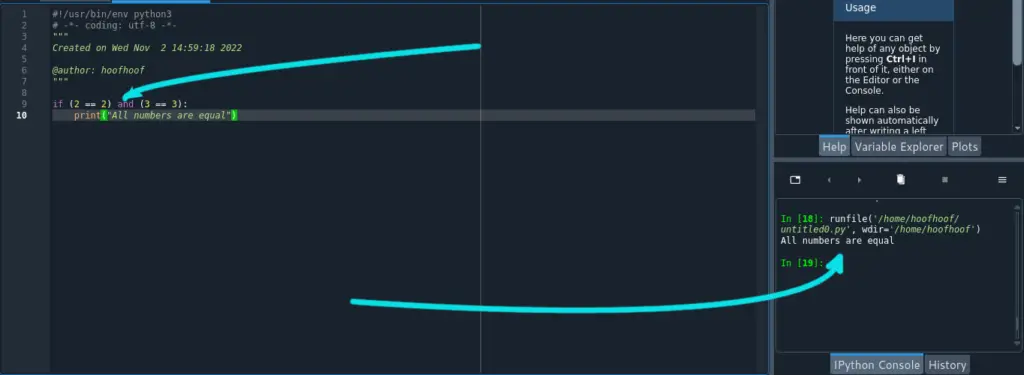
It can be confusing and very easy to mix up the syntax when you are simultaneously learning more than one programming language.
&& and AND operators can be easily mixed up when you are working with Python and JavaScript code at the same time.
It’s true that the && and AND operators can be easily mixed up, especially when you’re working with both Python and JavaScript code simultaneously.
While the operators serve a similar purpose in both languages, their syntax and usage differ.
Let’s see how the operator work or not work in both programming languages:
Python:
In Python, the logical AND operator is represented by the ‘and’ keyword.
It evaluates two expressions and returns True if both expressions are true; otherwise, it returns False.
Here’s an example code in Python:
x = 5 y = 10 if x < 10 and y > 5: print("Both conditions are true.") else: print("At least one condition is false.")
Output
Both conditions are true.
So, in short, there is no && operator in Python.
On the hand, for
JavaScript:
In JavaScript, the logical AND operator is represented by the && symbol.
Similar to Python, it evaluates two expressions and returns true if both expressions are true; otherwise, it returns false.
Here’s an example JavaScript code:
let x = 5; let y = 10; if (x < 10 && y > 5) { console.log("Both conditions are true."); } else { console.log("At least one condition is false."); }
Results:
Both conditions are true.
Here’s where the mixing of the two operators comes in,
Mixing up the operators:
When working with both Python and JavaScript code simultaneously, it’s easy to inadvertently use the wrong operator in the wrong language.
For example, mistakenly using the && operator in Python or the ‘and’ keyword in JavaScript can lead to syntax errors.
Python example with incorrect usage of JavaScript’s && operator:
x = 5 y = 10 if x < 10 && y > 5: # Incorrect usage of && print("Both conditions are true.") else: print("At least one condition is false.")
Output:
SyntaxError: invalid syntax
JavaScript example with incorrect usage of Python’s ‘and’ keyword:
let x = 5; let y = 10; if (x < 10 and y > 5) { // Incorrect usage of 'and' console.log("Both conditions are true."); } else { console.log("At least one condition is false."); }
JavaScript code output:
SyntaxError: Unexpected identifier
To avoid mixing up the operators, it’s crucial to be mindful of the specific syntax and rules of each programming language.
Paying close attention to the language you’re working with and using the correct operator accordingly will prevent syntax errors and ensure your code functions correctly.
But with time and practice, identifying these syntax errors should be pretty much easy as you write your code.
As you have seen, you only need to know that && is not a valid syntax in Python. Instead, && operator is replaced by the ‘and’ keyword in Python.
How to avoid confusion between && and AND in Python
In the beginning, it can be hard not to confuse the two, especially when learning logical operators in theory, such as in a Discrete Mathematics course.
Fortunately, you can avoid confusing && operator and AND keyword in Python by:
- Practicing a lot and writing logical-AND operations in Python
- Focusing on learning one programming language at a time if you are a beginner in all of the programming languages. The goal is to avoid syntax confusion across different programming languages, such as Python and JavaScript.
- Using a code editor such as Visual Studio Code or IDE such as Pycharm to write your code. An IDE or code editor will help you highlight your code’s syntax errors as you write.
Related Questions
What is the difference between && and AND in Python?
In general programming, && and AND are the same in implementation. && and AND are used to perform logical operations that yield logical value TRUE if both statements are equal.
Otherwise, the logical operations will be FALSE if the statements are not equal.
The difference between the && and AND arise when used in Python programming language. You cannot use && in Python because it is not a valid syntax.
However, you can use ‘and’ to perform logical AND operations in Python.
Thus, an AND boolean operator in Python can be used to test conditions and/or perform logical operations and test whether there is a relationship between phrases or statements.
What happens if I mistakenly use && instead of ‘and’ in Python?
If you mistakenly use && instead of ‘and’ in Python, you will encounter a syntax error.
Python does not recognize && as a logical operator, so it will raise an error and prevent your code from executing properly.
It is important to use the correct syntax to avoid these errors and ensure the desired functionality of your code.
Python uses the ‘and’ keyword as the logical AND operator instead.
Error message:
When you attempt to use && in your Python code, you will receive a SyntaxError. Python’s interpreter will raise this error because it expects the ‘and’ keyword instead of &&.
Example:
Consider the following example where I mistakenly use && instead of ‘and’:
# Incorrect usage of &&
x = 5 && y = 10
When you try to run this code, you will see an error message similar to:
SyntaxError: invalid syntax
How to fix it:
To correct the mistake, you need to replace && with ‘and’ in your code.
Here’s the corrected version of the previous example:
# Corrected usage with 'and'
x = 5 and y = 10
By using ‘and’ instead of &&, you ensure that your code follows the correct syntax in Python.
Comparison between && and ‘and’:
Operator | Usage in Python | Result |
---|---|---|
&& | Incorrect | Raises a SyntaxError (invalid syntax) |
‘and’ | Correct | Evaluates the logical AND operation correctly |
It’s important to remember that Python’s syntax follows a specific set of rules, and using the correct operator is essential for your code to run without errors.
By understanding the consequences of mistakenly using && in Python and the correct usage of the ‘and’ keyword, you can avoid syntax errors and ensure your code functions as intended.