If you are a programmer or someone who has never written code, you must have noticed the weird spaces in many programs’ code. Some of these spaces may be whitespace or tab that remain consistent across the whole program code.
If you are like me, before I knew how to code, you may have asked why programmers love to indent their code.
Programmers indent their code to enhance code readability, improve code organization, separate code with different functionality into blocks, separate functions, fulfill a programing language requirement, and structure nested code.
When going through well-written and spaced code, I bet it was easier for you to scan through the program and see how the code is structured. Moreover, understanding the functionality of each block of code was way much easier. One of the advantages of adding spaces to the code is readability.
But, before we dive into why programmers love to indent their code, what is indentation?
Indentation is adding horizontal whitespaces in your code to improve readability, enhance code structuring, and in some programming languages, fulfill the syntactical correctness of the code.
Reasons why programmers indent their code
1. Indentation improves code readability
Adding indentation to your code improves code formatting, making it scannable to human readers. The extra spaces make the code visually appealing and not some mumble jumble of code.
With indents, it is easier to follow the code structure, making the identification of each code block easier. As each code block has different statements, it is easier to identify the functionality that these blocks contribute to the overall program.
2. Indentation is required in some programming languages
In some programming languages, such as Python, indentation is required when writing code for functions, classes, conditional statements, iteration, e.t.c.
Without the indentation, these programming languages will throw IndentationError. Thus, the code won’t compile successfully until you correct the indentation error.
Here is an example of a code implemented in Python that would result in an Indentation Error.
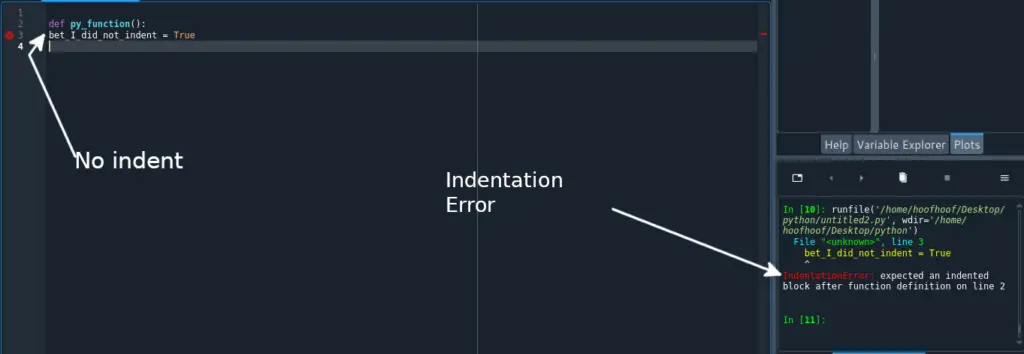
3. Add indentation to enhance code organization
Indentation helps achieve code organization and separate functionality that is different for each code block. You can use indents to separate code within control flow structures, functions, objects, selection statements, e.t.c.
For example, see how each of the following JavaScript codes looks great with indentation:
var myObject = {
"name": "Object Name",
"id": "2"
}
function walk(person_to_walk, person_commanding){
alert(person_commanding + " : Walk the plank, me hearties!");
alert(person_to_walk + " : I am walking, geez!");
}
walk("Steve (Having planned a myutiny)", "Captain");
In the code above, notice how the code will look disorganized without the indentation. You wouldn’t know where the object and the function code start and end.
The code below will still give the same results, but it looks disorganized.
var myObject = {
"name": "Object Name",
"id": "2"
}
function walk(person_to_walk, person_commanding){
alert(person_commanding + " : Walk, dude!");
alert(person_to_walk + " : I am walking, geez!");
}
walk("Steve (Having planned a myutiny)", "Captain");
You can use indentation to better organize your code and separate code blocks that may look the same but have different functionalities.
For example, adding indents is a great way to separate functions that perform different tasks in a program. Besides, where functions are nested together, it is very easy to differentiate the hierarchy between them.
4. Adding indents makes debugging easier
Adding indentation helps code debugging easier both when using a program to highlight errors and scanning through your code by yourself.
When using an IDE or code editor to debug your code, identifying errors in the code is easier when each statement is in its own line. Otherwise, you would have too many errors in one line, and the IDE/code editor shows debugging results in that one line.
When scanning your code for syntactical errors, it is much easier when everything is organized into code blocks. One great way to achieve code organization is to add indents and whitespaces.
5. Indentation prevents writing messy code
When everything is in one line, there is a high chance that you will write messy code, especially on hierarchical statements.
Using one line to write nested tags will usually result in some tags not being closed or following the order intended.
6. Indents can be used to show multilevel/nested code
Functionality that is implemented in the form of nesting such as nested tags, tables, lists, e.t.c; it is much easier to use indents to code and show each level.
Using one line to code an entire table or list would result in a very messy and not-so-easy-to-debug code.
7. Indentation helps build consistency in your code
Using indentation across your program code helps build consistency. Consistency is an important code structuring factor that helps save time when revisiting your code base.
When you revisit your code that has a consistent separation of declarations and functionalities, it is easier to identify these code blocks by looking at the indentation.
Besides, revisiting a nested code is much faster to scan through- saving time, as you do not need to go through each level. As each nested tag has its own indentation level, it is much easier to identify a hierarchy based on levels.
8. Indentation helps fulfill PEP 8 whitespace ethics
PEP 8 whitespace ethics require adding indentation to every new iteration of blocks, such as for loops, while loops, e.t.c.
Here’s an example of adding indentation to an iteration from a for loop in Python:
# adding indentation to every new iteration as per PEP 8
for i in range(2):
print("first iteration")
for j in range(3):
print("second iteration")
print(f"i = {i}, j = {j}")
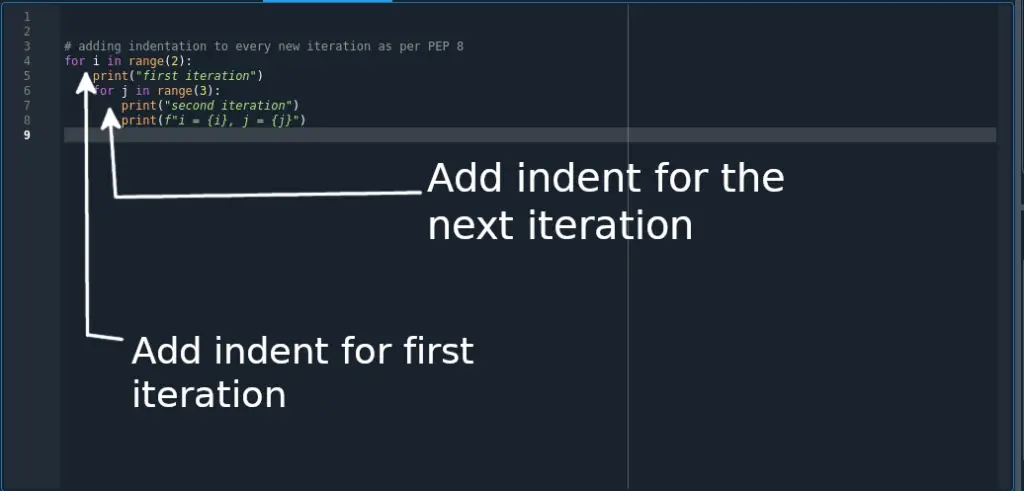
Common questions
Is indentation required in Python?
Indentation is required in Python not as a styling requirement but for syntactical correctness. For python code to compile successfully, some code blocks must have an indentation. These code blocks are for loop, while loop, functions, classes, if conditional, switch statement, e.t.c.
Here is an example of how to use indentation in for loop, function, and a class block in Python:
# for loop
for i in [1, 2, 3, 4, 5, 6, 7, 8, 9]:
print(i)
# function block
def main():
name = input("Name: ")
preferred_lang = input("Preferred Programming Language: ")
programmer = Programmer(name, preferred_lang)
print(f"{programmer.name} prefers to code in {programmer.preferred_language}")
# class block
class Programmer:
def __init__(self, name, preferred_language):
self.name = name
self.preferred_language = preferred_language
main()
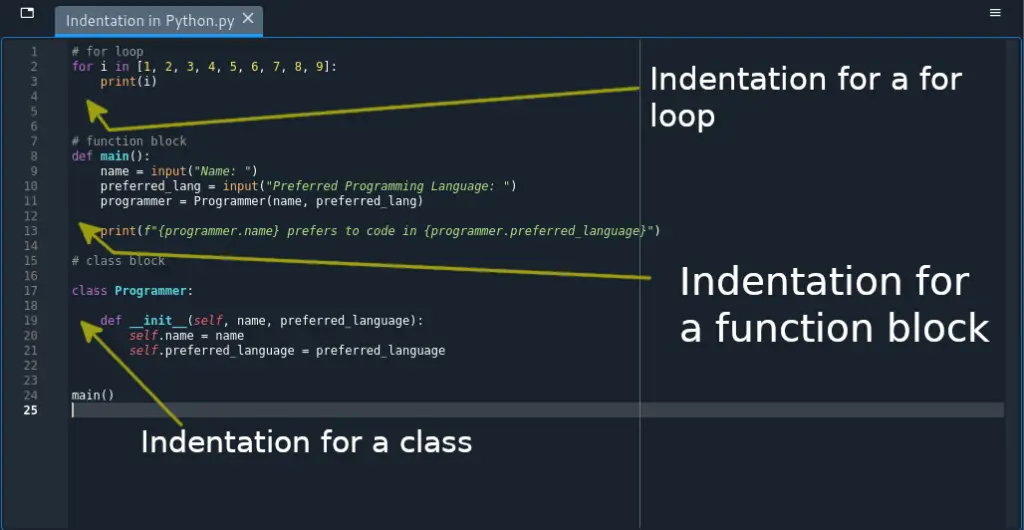
So, as a requirement, you must indent your Python code when declaring some code blocks such as function or class blocks. Otherwise, your Python code will not be interpreted correctly and thus not execute successfully. If you do not insert an indent into such a block, you will get an indentation error when executing your program.
Does indentation matter in HTML?
For human readers, indentation does matter in HTML. However, indentation does not matter in HTML for browsers or applications that understand markup language.
I bet you have heard of HTML minification. Minification works by stripping off the extra whitespace and indentation in HTML code to minimize the file size. Thus, indentation won’t matter in this case because the browser does not require HTML to have whitespaces.
When should you indent in HTML?
You should indent your HTML code during development. However, you may strip off extra spaces in your code when deploying your website to save on space and improve page load speed.
Therefore, when writing code, you should indent your HTML code and later use code minification to remove all the extra space in your HTML and CSS files.
If you are going to use the following tags in your HTML tags, it is a good practice to indent:
How many spaces are required for an indent?
For most programming languages, an indent that is 2 or 4 spaces is ideal.
How to indent your code?
Indenting your code is a very simple process that involves using the space bar or the tab key. When inserting an indent with the tab key, you should only press it once, and it will automatically add 4 spaces indent in your code.
On the other hand, you must press the space bar twice or four times to insert 2 or spaces indent in your code.
How to indent your code in Visual Studio Code?
Indenting your code in Visual Studio Code is a straightforward process involving using the tab key, spacebar, or pressing CTRL + ]. You may use any of the three methods on a blank line, but when you want to indent a line with code, you can only use the tab key and the CTRL + ] combination. If you highlight part of the code you want to indent and use a space bar, you will end up deleting your line of code.
How to indent existing code in VS Code
Step 1: Highlight the code you want to indent
Step 2: Press the tab key to add a forward indent. You may use the SHIFT + TAB key to remove an indent. Alternatively, you may insert a new indent with the CTRL + ] combination. To remove indentation in your highlighter code, press CTRL + [.
Conclusion
Indentation is essential to writing code because it helps achieve code organization and structuring. Programmers love to indent their code to improve code readability, organization, and separation. In some programming languages, such as Python, it is a requirement to indent your code to avoid compilation errors.
Besides, indentation makes debugging process and scanning through your code easier.
That’s it for this article.
Crack on, Dev!