As a programmer, generating random unique numbers or values is essential, especially when working with cryptography, computer simulations, generating statistical data, creating encryption keys, selecting random samples from large data sets, creating tokens, e.t.c.
One language to start with when learning how random numbers are generated is Python. As you know, Python has a reputation for its syntax being easy and more beginner friendly. Therefore, learning how to generate these random numbers should be easy.
You will not sleep in class.
Right?
Well, in this article, let’s see how you can create random numbers from a range of numbers. Also, we’ll see how to choose random data from a set of data, such as a list.
How to generate random numbers in Python
Generally, there are a couple of ways that you can generate random numbers in Python. Here are the methods used in Python to generate random numbers.
- random ()
- uniform()
- shuffle()
- choice()
- randrange()
- randint()
- sample()
- randbelow()
Most of the random number generators are found in the random module.
Let’s see how each method generates random numbers between 1 and 100.
How to use random() function to generate numbers between 0 and 1
The random() function is used to generate random floating point numbers without specifying a range in its arguments. Thus, it automatically creates random floats between 0 and 1 without taking any arguments. If you try to supply arguments to the random() function, it will throw a TypeError error.
Here’s how random.random() function works.
First, import the random module:
import random
Generate float value between 1 and 100 using this line of code:
random_float_num = random.random()
Results
print(random_float_num)
0.970792463262149
After a couple of iterations, I was able to generate these random floats
0.8194667995488517
0.9008248489634068
0.43750645739092076
0.5571071809069698
0.6812507610649364
...
See! Generating random numbers using the random() function is as easy as that.
How about storing these random values in a list?
But captain! Can’t we keep the lesson for tomorroooow?
Well, me hearty, no!
Heave ho!
Back to storing randomly generated floating point numbers in a list in Python topic.
We can use a for loop, where each iteration will create a unique number and append it to the list.
my_list = []
for i in range(10):
my_list.append(random.random())
print(my_list)
Run the program, and the results should be
[0.23102307652305298, 0.7060600649733757, 0.9041601515093399, 0.2917686367139777, 0.8707888721578918, 0.5636716004414297, 0.6241793430749701, 0.5920495457033083, 0.05038907958960204, 0.7913687702087498]
Your numbers should be unique; every time you execute the program, these numbers will change.
Enough of the random.random() function. I almost forgot there are other ways to generate random numbers in Python.
uuuuh! See who is smiling
How to generate random floating point numbers between 0 and 3 using uniform() function
We can use the random() function to generate random floats. However, the random() function only works with a range between 0 and 1.
We can use the uniform() function to generate random floating point numbers out of the range of 0 and 1. Thus, with a uniform() function, you can generate random floats between 0 and 3 or 5 to 10.
Let’s see how uniform() function works:
As usual, import random module
import random
Generate a random float value given a range, 0 and 3
my_num = random.uniform(0, 3)
Output
print(my_num)
1.063248889096625
2.7633614142989966
2.672820913111723
1.5005262176533103
2.6311606375956065
1.765827279297373
2.6829910434686592
0.49271805553734827
1.2567870822944995
2.7929604206487046
1.6551360352814077
How to use randint() function to generate numbers between 1 and 100
We have seen how to create random floating point numbers between 0 and 1. How about generating random numbers between 1 and 100?
To generate random numbers between 1 and 100, we can use the randint() function that takes two arguments. The first argument is the starting number, and the second is the last number of the range you want to generate numbers from.
Here’s how it works:
Import the random module
import random
Generate random numbers between 1 and 100
random_num_b_1_100 = random.randint(1, 100)
Results
print(random_num_b_1_100)
30
56
78
34
57
Voila!
We have a couple of randomly generated numbers between 1 and 100. The randint() function takes one step to generate the numbers. Thus, you can have 31 and then 32 in your random numbers following each other.
How to generate random numbers between 10 and 100 using randrange() function
Sometimes, you may want the randomly generated numbers to be multiple of 2. In this case, you can use the randrange() function that takes a third argument that defines the interval between randomly generated numbers.
For our case, we need to generate only even numbers. Thus, we can use an interval of 2.
Here’s how it works:
Import the random module
import random
Generate random numbers that obey an interval of 2
random_num_step_2 = random.randrange(2, 100, 2)
Output
print(random_num_step_2)
30
52
80
46
42
...
How about generating even numbers between 100 and 1000
You can use the randrange() function to generate numbers randomly following an interval of two. But the starting number must be odd.
random_num_step_2 = random.randrange(101, 1000, 2)
The code above generates random odd values between 101 and 1000
How to use sample() function to create a list of random numbers from 1 to 10
All the methods we have seen generate random single values. When you want to create multiple random numbers at once in Python, you can use the sample() function.
The sample function takes two arguments:
- The population within which to create random numbers from. Here, you can give a range of numbers, another list of numbers, e.t.c.
- The second parameter defines how many numbers you want to generate from your population.
Here’s how it works
Import the random module
import random
Create a list of random numbers using the sample() function. Also, you must define the population and the number of random numbers to generate.
my_list_of_random_nums = random.sample(range(1, 10), 6)
Output
print(my_list_of_random_nums)
[9, 8, 1, 4, 7, 5]
[9, 7, 3, 8, 1, 2]
[2, 1, 9, 4, 3, 6]
You should take care not to specify more numbers from a sample population that is less.
As another example, let’s generate a list of random numbers without duplicates between 1 and 100:
import random
list_of_numbers_b_1_100 = random.sample(range(1, 100), 50)
print(list_of_numbers_b_1_100)
# results
[88, 37, 25, 20, 45, 55, 38, 95, 58, 53, 69, 27, 49, 28, 90, 99, 36, 35, 46, 50, 18, 68, 91, 86, 10, 71, 96, 80, 22, 3, 67, 79, 94, 59, 93, 54, 2, 43, 52, 82, 61, 81, 92, 39, 42, 97, 16, 14, 13, 15]
How to use shuffle() function to generate random numbers or values from a list
shuffle() function can be used to randomly rearrange numbers or values from a given list in your Python code. Therefore, you can create random scenarios on which number or who is number one, second, and so on.
Let’s say you have a list of numbers or names of people
list_of_numbers = [1, 2, 3, 4, 6, 7, 8, 9, 10]
list_of_players = ["Steve", "Penelope", "Mary", "Jack", "Wambui", "Cate"]
You can randomly rearrange these numbers or people with the occurrence of a number or person in a particular location not predetermined.
random.shuffle(list_of_numbers)
random.shuffle(list_of_players)
Results:
print(list_of_numbers)
print(list_of_players)
[2, 7, 8, 6, 10, 9, 4, 1, 3]
['Cate', 'Penelope', 'Jack', 'Steve', 'Wambui', 'Mary']
[10, 2, 4, 7, 8, 9, 3, 6, 1]
['Jack', 'Steve', 'Mary', 'Wambui', 'Penelope', 'Cate']
This can be useful when you are with your friends at a wedding struggling to get one of the rarest and mouth drooling food and you don’t know who goes first.
You: (staring at me. Looking like you don’t get it.)
Me: You know, food that messes with your medulla oblongata and makes you drool.
You: [pauses] Huh!
You: Wait! (Making a facial expression that makes you look like you have just realized something you never knew )
Steve, you do mouth water, and drool. Like Rick from (Rick and Morty show). Sorry Rick.
Me: Nooo!
Well, probably. [pauses and thinks] When I see alluring, adorable, bewitching, and beauteous girls.
But!
That’s no the point!
You: Laughing and repeating the statement: You do drool, mate?
We were talking about random numbers and people.
Froom a… a… a… list
Right?
You are getting distracted , Morty!
Focus!
So, you can use the shuffle() function to generate random rearranged positions of numbers or items in a list.
How to use choice() function to choose a random number or item from a sequence
If you have a list of numbers, items, or people, you can choose a random item/person/number from the list using the choice() function.
Here’s how it works:
Let’s say you have a list of people and want to choose a random person to be a representative.
list_of_candidates = [
'Olivia',
'Emma',
'Charlotte',
'Amelia',
'Ava',
'Sophia',
'Isabella',
'Mia',
'Evelyn',
'Harper',
'Luna',
'Camila',
'Gianna',
'Elizabeth',
'Eleanor',
'Ella',
'Abigail',
'Sofia',
'Avery',
'Scarlett',
'Emily',
'Aria',
'Penelope',
'Chloe',
'Layla',
'Mila',
'Nora'
]
You can choose a random candidate using the random.choice() function:
winner = random.choice(list_of_candidates)
The result is one candidate chosen randomly from the list of candidates:
print(winner)
Penelope
How to use randbelow() function to generate numbers between 0 and 9
A new secrets module introduced in Python 3.6 offers randbelow() function that is better suited for cryptography and security. Therefore, as per Python Org, the secrets module is better suited for generating secret information such as tokens, passwords, e.t.c.
You can generate random numbers in Python without using random by using the secrets.randbelow() function.
The randbelow() function can be used to generate random numbers between a range of 0 to 9. The random numbers are inclusive. Thus, you will get a 0 or 9 in your random numbers.
Here’s how it works:
Import the secrets module
import secrets
Generate random numbers using the randbelow() function
secrets.randbelow(10)
Note that randbelow() is much slower than randrange() when generating random numbers:
import timeit
print(
'randbelow() takes:',
timeit.timeit("secrets.randbelow(10)", setup="import secrets"))
print(
'randrange() takes:',
timeit.timeit("random.randrange(10)", setup="import random"))
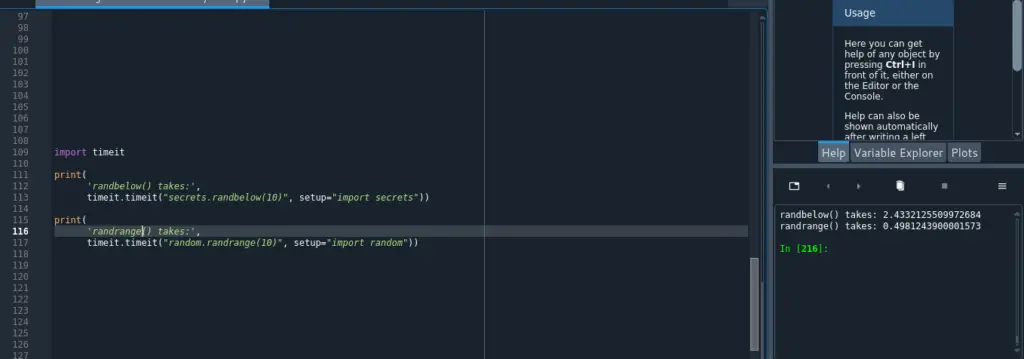
[Summary]
The table below shows how to generate Python’s random numbers and values from 0 to 1, 1 to 10, 10 to 100, 100 to 1000, and 1000 to 10000.
Generate a random number between/from | Python code used |
0 to 1 | import random random.random() |
0 to 3 | import random random.uniform(0, 3) |
0 to 6 | import random random.uniform(0, 6) |
0 to 9 | import random random.randint(0, 9) -OR- random.randrange(0, 10) if you want a floating-point number random.uniform(0, 10) |
0 to 10 | import random random.randint(0, 10) |
1 to 10 | import random random.randint(1, 10) |
1 to 5 | import random random.uniform(1, 5) |
1 to 100 | import random random.randint(1, 100) |
Generate a list of random numbers between 1 and 100 | import random random.sample(range(1, 100), 50) |
Generate negative random float from negative 1 and 1 | import random random.uniform(-1, 1) |
Generate a negative random number from -1 and 1 | import random random.randrange(-1, 1) -OR- random.randint(-1, 1) |
1 to 6 | import random random.uniform(1, 6) |
1000 and 9999 | import random random.randint(1000, 9999) |
Related Questions
How do you generate 20 random numbers in Python?
You can generate 20 random numbers in Python by using the random.sample() function. The sample() function allows you to specify the number of random values to choose from a population of numbers.
Here’s the code for generating 20 random numbers between 1 and 100 in Python:
import random
my_list_of_random_20_nums = random.sample(range(1, 100), 20)
print(my_list_of_random_20_nums)
Generate 30 random numbers in Python.
import random
my_list_of_random_30_nums = random.sample(range(1, 100), 30)
print(my_list_of_random_30_nums)
How do you generate 10 random integers in Python?
Here’s the code to generate 10 random integers in Python
import random
my_list_of_random_10_ints = random.sample(range(1, 100), 10)
print(my_list_of_random_10_nums)
That’s it for this article.
Continue practicing, and it will become second nature to you.
Heeeeave!