When writing programs, programmers choose various default and custom names for their variable names, keywords, functions, classes, modules, e.t.c.
The main goal is to use names that are meaningful and describe what each specific line of code or code block is intended for. Thus, they create variable names and use default keywords that make sense to what they represent.
The reason is to make their code executable, readable, and easier to understand when they revisit their code later, or someone else looks at their code.
More often, some custom functionality may collide with functionality best described with a keyword already used by default in Python or is a reserved word. Thus, you and software engineers may find yourself using identifiers for your code blocks that may conflict with other default or module identifiers.
Thus, the objective of this article is guided around the question: Can a keyword be a variable name in Python?
First, let’s understand what keywords, identifiers, and variables used in Python are.
What is a keyword in Python?
Keywords are predefined, specific, and reserved words that hold special meaning or functionality in Python. Therefore, to use or implement some functionality, such as creating a new Python function, you would use a specific keyword to produce the functionality or code you want.
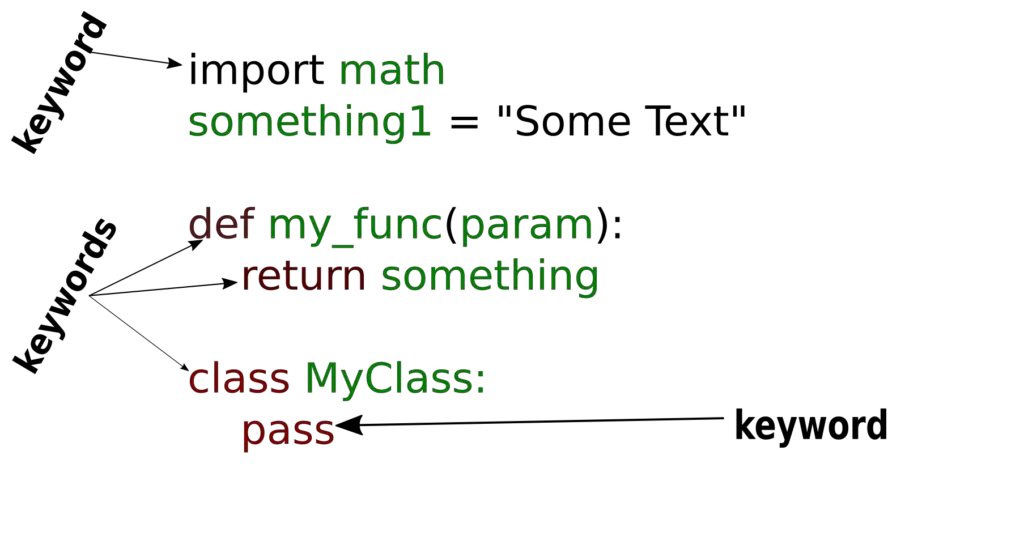
For our function declaration functionality example, you would use the def keyword to create a new function in Python.
Another example of a common Python keyword is the print keyword. The keyword print has a special and reserved functionality used to produce textual output to the screen in Python.
In simple terms, what are keywords in Python? Keywords are the default and already declared identifiers that are used to refer to specific functionality used in Python.
Just as identifiers, keywords also obey declaration rules. When declaring these keywords, Python developers and maintainers follow the same rules of using letters in uppercase and lowercase, digits, and underscores.
Thus, keywords can have letters, numbers, and underscores.
Let’s see the rules around using keywords in Python.
But first, why are there rules for keyword use in Python and other programming languages in general? Python declares some specific words not to be redefined or used in your code to avoid conflicting entity identifiers. These words are the default keywords used in Python and the reserved words.
The goal of reserving these words is to
- Avoid conflicting names between default identifiers, i.e., keywords and reserved words with the user-declared identifiers used in function variable names, classes, and modules.
- Avoid programming language compromises that arise from using reserved words known to the compiler. Thus, the compiler may terminate prematurely when it encounters the same words with different functionalities and code.
For that reason:
- You cannot use keywords in your identifiers, i.e., variable, function, and class names.
- Reserved words should not be used to name your customer identifiers.
Therefore, you should know the common words used as Python keywords to avoid using them to name your variables, functions, modules, classes, e.t.c.
Here is a list of keywords in Python that cannot be used as identifiers
break | in | import | None | True |
raise | yield | nonlocal | def | False |
is | lambda | global | class | return |
except | del | finally | from | pass |
continue | assert | with | try | as |
while | for | else | elif | if |
not | or | and |
The table above shows a list of keywords you cannot use to name your variables, classes, functions, modules, e.t.c.
Reserved words in Python
What are reserved words? Reserved words are a number of words that Python reserves for designated functionality. Thus, you cannot use these keywords as identifiers. These words can never be used to name variables, function names, class names, modules names, e.t.c. Keywords are reserved words in Python.
Here is a list of all the 33+ reserved words (as per Python 3.10) in Python that cannot be used as identifiers in your Python code.
False | class | from | or | None |
continue | global | pass | True | def |
if | raise | and | del | import |
return | as | elif | in | try |
assert | else | is | while | async |
except | lambda | with | await | finally |
nonlocal | yield | break | for | not |
You should not cram all these words when you are learning Python. Instead, you should focus on practicing more; these words will be second nature to you.
If you unsure about a word that could be a Python keyword or reserved word, you may use the help utility to find all the reserves words in Python.
Here’s how to browse the list of reserved word used in Python.
Step 1: Open the Terminal (CTRL + ALT + T) or the command prompt
Step 2: Type ‘python’ and hit Enter
The Python interpreter should open and you should be able to issue Python commands.
Step 3: Type help(“keywords”) and press enter
Python should list all the reserved words. You should now be able to confirm that the word you want to use as an identifier is a reserved word or not.
What is an identifier in Python?
An identifier is a name given to a variable, function, class, module, or any other object. Identifiers are descriptive of the code they represent.
Another way to define an identifier is that it is a name that identifies or labels either a particular object or a class of objects. An ‘object’ can be created or represented by a variable, a function, a class, e.t.c. Thus, an identifier is a term given to a value of a variable, a label of a class, or a label of a function.
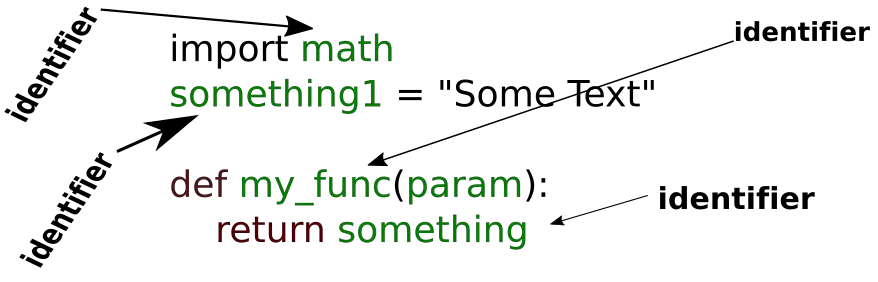
From the image above, it is clear what an identifier in Python is. Identifiers in Python identify a variable, e.g., something1; a module, e.g., math; a function, such as my_func; or a class, such as MyClass, ListView, and BlogPost.
As seen from the examples above, identifiers in Python are user-defined. Thus, they are defined by a module’s maintainers or developers. For example, the programmer or organization which wrote the math module decided to identify the module with the name math.
So, an identifier is used to refer to a value. Thus, an identifier gives an entity a name.
However, when naming identifiers, you, other programmers, and module creators follow a naming rule that obeys specific rules. These rules for naming the identifiers include:
- Names must contain a sequence of letters, digits, or underscores. Thus, an identifier must have its first character, either a letter, digit, or underscore. For example, valid variable names are myvar, var1, and _var_with_an_underscore.
- Identifiers must not conflict with default Python keywords and reserved words. Thus, identifiers must be unique and should not have similar spelling and case for any other Python keywords.
- You must separate the words with an underscore when using more than one word for your identifier. Here are examples: my_variable, test_speed, article_title, e.t.c.
- You may use any case for your identifiers. Thus, Student, STUDENT, subject, and hard_worker are examples of valid identifiers.
When creating an identifier, just remember that
- It can have numbers (0-9)
- It can contain uppercase letters (A-Z)
- An identifier can have lowercase letters (a-z)
- It can start or have an underscore (_)
- An identifier should always start with a non-numeric character
- An identifier should not contain numeric characters only. Thus, 2347, 45, and 59 are not valid identifiers.
- Identifiers are case sensitive
- We cannot use Python keywords as identifiers
- An identifier has no limit to the name length
A variable is a kind of an identifier in Python. Other kinds of identifiers are function names, class names, modules, e.t.c.
What is a variable name in Python?
A variable name is a label or term used to reference a reserved computer memory location, which in general terms, is called a variable. So, a variable is a reference term for a variable.
There is a difference between a variable and a variable name.
A variable is a reserved computer memory location that is used to store values that the program may require as input for manipulation. These values can be integers, long integers, floats, and strings. Thus, a program requests this data stored in the memory location of the computer for processing or supporting the core operations of the Python program.
Variable names easily refer to memory addresses in a computer storing variables, i.e., integers, long integers, strings, and floats.
As a programmer, it would be so hard to reference the actual memory address in your Python code. An example of a memory location in hex format is 0x7f6835c88170.
So, instead of writing such a large hex number, programmers utilize variable names to label such a memory address in their code easily. For our hex example, I could easily reference the address location with a variable name x.
See the code below
x = 5
print(hex(id(x)))
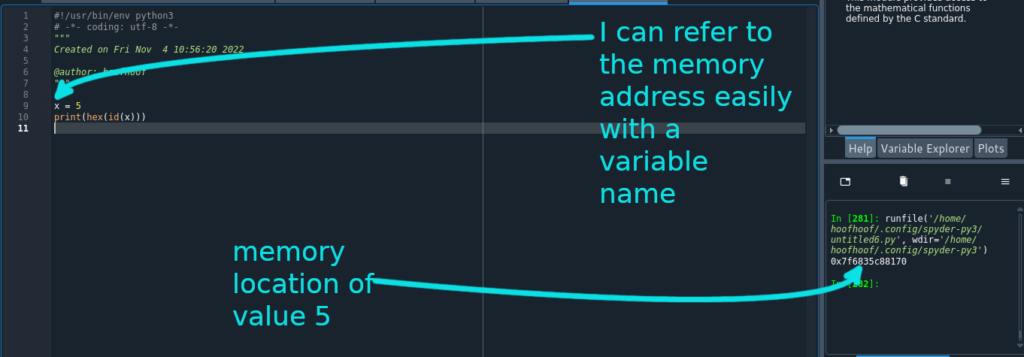
As you have seen, variable names are also labels that refer to ‘objects’, variables. Therefore, a variable name is a kind of identifier. For that reason, the same rules that are followed when naming identifiers also apply when declaring variable names.
In summary, here are the most common rules of naming variables that you will most likely encounter:
- Python accepts only alphabets, underscores, and digits for naming variables. Variable names can only have letters in uppercase and lowercase, digits, and underscores.
- To name your variables, you can use alphanumeric characters (letters and digits) and underscores. Examples of valid variable names are article, my_var, var2, _var1, and my_var_with_underscores.
- You cannot use spaces in your variable names. Spaces are not permitted when naming your variables. Examples of invalid variable names are my var, var with spaces, var 1, e.t.c.
- Variable names may not start with a digit. Thus, 1var, 2var, 3x, 2y, e.t.c, are not valid for a variable name.
- The first letter should be a letter or an underscore.
- No two variables should have the same name. Each variable name must be unique in your Python program.
Now that you understand the rules of naming your variables let’s see how you can create a variable name in Python.
In Python, you declare a variable the moment you assign a value to it. To assign a value to a variable, you must use an equal sign to do that. Here is the syntax:
variable_name = value
my_var = 10
my_var is an example of a variable name that references the value 10 in the computer’s memory.
As you have noticed, you can join multiple words used in a variable name using underscores. So, if your descriptive name for a variable fits more than one word, use underscores to join them.
Another example of a variable using underscores is is_student, speed_limit, variable_y, e.t.c.
Types of data that variable can hold
- Integer, e.g., 1
- String, e.g., ‘Penelope‘
- Float, e.g., 3.45
- Long integer, e.g., a very long number with too many figures
Generally, you should not use special characters, single numbers, and non-descriptive texts to name your variables in Python.
What is the difference between keyword and identifier in Python
The differences between keywords and identifiers in Python are
Keyword | Identifier |
Can contain only alphabetical characters | It can consist of alphabetical characters, numbers, and underscores |
Keywords are predefined | The programmer can create identifiers |
Keywords are reserved for unique functionality | Words used as identifiers are not usually reserved for unique functionalities. |
Keywords hold special meaning to Python. | Identifiers are labels given to variables, functions, or classes |
Keywords mostly start with small/lowercase letters | Identifiers can start with a letter that can be in uppercase or lowercase. Besides, it can also start with an underscore. |
Keywords mostly contain only alphabetical characters. | An identifier can contain digits, alphabetical characters, and underscores |
There are no special characters or symbols used for keywords | You cannot use special symbols in your identifiers except an underscore. |
Examples of keywords are def, pass, class, if, is, in, and, return, import, e.t.c. | Examples of identifiers are my_var, var1, Article, ListView, speed_limit, student_name, subject_title, e.t.c. |
What is the difference between keywords and variables
Here are the differences between keyword and variable names in Python
Keywords | Variables |
Usually declared by programming language maintainers/developers. Keywords are predefined. | Usually declared by the programmer. |
Keywords cannot be used as variable names. Keywords are uniquely identified with their functionality in Python. | Variables names must be unique. |
Keywords cannot be classified into various groupings. | Variable names can be classified into local and global variables. |
What is the difference between a variable name and an identifier
Variables and identifiers differ from each other in the following ways.
Variable | Identifier |
A variable is used to hold a value | An identifier identifies an entity that is unique in a program |
A variable is a name given to a memory location | Identifiers uniquely refer to a program entity at the time of execution |
A variable is an identifier | Some identifiers are not variables. |
Examples of variables are integers, floats, and strings. | Examples of identifiers are Subject, number2, is_ripe, is_valid, etc. |
Can a keyword be a variable name in Python?
In Python, a keyword cannot be used as a variable name or identifier. Therefore, you should not use a Python keyword to name your program’s variables, functions, classes, or modules.
Here are the reasons why we can’t use a Python keyword as a variable name:
- You will find that you cannot use keywords. They are already reserved words in Python.
- Keywords are unique and hold special functionality in Python. Thus, overriding the keywords with new functionalities will lead to compilation and execution compromises/errors.
- You will end up with conflicting names that will have problems in your program.
If you apply all the rules of naming identifiers discussed above, a keyword cannot be a variable name in Python. Similarly, the same principles apply to other programming languages.
So, the verdict is that you can’t use it as a variable name. Python keywords cannot be used as variable names.
That’s it for this article. With the first iteration, it may be hard to understand which is which, especially identifiers and variables. But, after some practice and reading the article a few times, it should click.
All right.
Heave ho!