In programming, environment variables play a crucial role in configuring and customizing applications, especially with web frameworks such as Django.
As a Python programmer, understanding how to access and utilize environment variables can greatly enhance your ability to create flexible and adaptable software.
Whether you are developing web applications, managing database connections, or working with API keys, accessing environment variables in Python allows you to harness the power of dynamic values that influence program behavior.
But how do you access environment variables in Python?
To access environment variables in Python, you have several options. The os.getenv()
function allows you to retrieve a specific variable, while os.environ
provides a dictionary-like object with all variables. Alternatively, you can use third-party packages like Python Decouple, Django-Environ, or Python-Dotenv for more advanced functionality.
These tools simplify the process of managing and accessing environment variables, making it easier to configure your Python applications across different environments.
Let’s look at each option and see how you can access environment variables in a development and production environment while referencing Django as context.
How to get an environment variable in a local development setup
In a local development setup, accessing environment variables is crucial for configuring your Python projects.
Fortunately, you have several options to retrieve these variables based on your specific requirements.
We will explore three common methods:
- Using
os.getenv()
, - Utilizing the
os.environ
dictionary and - Leveraging third-party libraries such as Python Decouple, Django-Environ, and Python-Dotenv.
How to use os.getenv() to access environment variables in Python
When working with environment variables in Python, one of the commonly used methods is os.getenv()
.
This function allows you to retrieve the value of a specific environment variable by its name.
Here’s how:
To access an environment variable using os.getenv()
, follow these steps:
Step 1: Import the os
module
Before using os.getenv()
, you need to import the os
module into your Python script.
This module provides functions for interacting with the operating system, including accessing environment variables.
import os
Step 2: Retrieve the value of an environment variable
To retrieve the value of a specific environment variable, call os.getenv()
and pass the name of the variable as a string parameter.
The function will return the value associated with that variable, or None
if the variable does not exist.
Here’s an example code retrieving the URL endpoint of a database common when connecting Python with a database such as PostgreSQL hosted on a remote server.
# Retrieve the value of the 'DATABASE_URL' environment variable
database_url = os.getenv('DATABASE_URL')
In the example above, os.getenv('DATABASE_URL')
retrieves the value of the 'DATABASE_URL'
environment variable and assigns it to the database_url
variable.
You can replace 'DATABASE_URL'
with the name of the environment variable you want to access.
Step 3: Utilize the retrieved value
Once you have retrieved the environment variable value, you can use it in your Python code as needed.
For example, you might use the value to establish a database connection or configure API credentials.
import os
# Retrieve the value of the 'API_KEY' environment variable
api_key = os.getenv('API_KEY')
# Use the retrieved value in your code
api_client.configure(api_key)
In this snippet,
os.getenv('API_KEY')
retrieves the value of the'API_KEY'
environment variable and assigns it to theapi_key
variable.You can then utilize the
api_key
value to configure an API client in your application.
Using os.getenv()
provides a straightforward approach to accessing environment variables in your Python programs.
However, keep in mind that if an environment variable does not exist or is not set, os.getenv()
will return None
.
Handling cases where an environment variable is not set or does not exist is crucial to ensure your code runs smoothly without encountering errors.
To handle such scenarios, you can use conditional statements or provide default values.
Here’s how you can handle these cases appropriately:
1. Using Conditional Statements:
You can use conditional statements, such as
if
orternary operators
, to check if the retrieved value fromos.getenv()
isNone
and take appropriate action.For example:
import os api_key = os.getenv('API_KEY') if api_key is not None: # Use the retrieved value api_client.configure(api_key) else: # Handle the case when the environment variable is not set print("API key is not set. Please configure it.")
In this example, if the
API_KEY
environment variable exists and has a value, it is used to configure theapi_client
.Otherwise, a message is displayed to indicate that the API key is not set, allowing you to handle the situation accordingly.
2. Providing Default Values:
Another approach is to provide a default value when retrieving the environment variable using
os.getenv()
.This ensures that even if the variable is not set, your code can continue to run with the default value.
Here’s an example:
import os # Retrieve the value of the 'API_KEY' environment variable api_key = os.getenv('API_KEY', 'default_value') # Use the retrieved value api_client.configure(api_key)
In this case, if the
API_KEY
environment variable is not set,os.getenv('API_KEY', 'default_value')
returns the specified default value,'default_value'
.You can replace
'default_value'
with the value that makes sense for your application.By incorporating these techniques into your code, you can handle cases where environment variables are not set or do not exist.
This ensures that your program gracefully handles such scenarios and avoids potential errors that could arise from using
None
values.Remember to tailor your handling approach based on the specific needs and requirements of your application.
More about handling existent and non-esistent environment variables here.
Remember, by leveraging os.getenv()
, you can easily retrieve the values of environment variables, enabling your Python applications to adapt to different environments and configurations.
How to use os.environ to retrieve environment variables in Python
In Python, the os.environ
dictionary provides a convenient way to access and retrieve environment variables.
To retrieve an environment variable using os.environ
, follow these steps:
Step #1: Import the os
Module
Before utilizing os.environ
, you need to import the os
module into your Python script.
The os
module provides functions for interacting with the operating system, including accessing environment variables.
import os
Step #2: Access the Value of an Environment Variable
os.environ
acts as a dictionary where the keys are the names of the environment variables, and the values are their corresponding values.
To retrieve the value of a specific environment variable, access it using square brackets with the variable name as the key.
# Access the value of the 'DATABASE_URL' environment variable
database_url = os.environ['DATABASE_URL']
In the example above, os.environ['DATABASE_URL']
retrieves the value associated with the 'DATABASE_URL'
environment variable and assigns it to the database_url
variable.
Replace 'DATABASE_URL'
with the name of the environment variable you wish to retrieve.
Note: If the specified environment variable does not exist or is not set, accessing it directly through
os.environ
will raise aKeyError
.Ensure that the environment variable you are accessing exists or handle this exception appropriately in your code.
Step #3: Utilize the Retrieved Value
Once you have retrieved the environment variable value using os.environ
, you can use it in your Python code as needed.
For example:
import os
# Access the 'API_KEY' environment variable from os.environ
api_key = os.environ['API_KEY']
# Use the retrieved value
api_client.configure(api_key)
In this snippet, os.environ['API_KEY']
retrieves the value associated with the 'API_KEY'
environment variable and assigns it to the api_key
variable.
You can then utilize the api_key
value to configure an API client or perform any other necessary operations in your application.
By utilizing os.environ
, you can easily access the values of environment variables in your Python programs.
However, keep in mind that directly accessing an environment variable through os.environ
without ensuring its existence may raise a KeyError
.
It is essential to handle this exception appropriately in your code to prevent potential errors.
To handle the possibility of a
KeyError
when directly accessing an environment variable throughos.environ
, you can use a try-except block to catch the exception and handle it gracefully.Here’s an example:
import os try: # Attempt to access the value of the 'API_KEY' environment variable api_key = os.environ['API_KEY'] # Use the retrieved value api_client.configure(api_key) except KeyError: # Handle the case when the environment variable is not set or does not exist print("The API_KEY environment variable is not set. Please configure it.")
In this example, the code tries to access the value of the
'API_KEY'
environment variable usingos.environ['API_KEY']
.If the variable exists and has a value, it is assigned to the
api_key
variable, and you can use it as needed.However, if the variable does not exist or is not set, a
KeyError
will be raised.By wrapping the code that accesses
os.environ
in a try block and catching theKeyError
in the except block, you can handle this exception appropriately.In the example above, the except block prints a message to indicate that the
'API_KEY'
environment variable is not set, allowing you to provide instructions or take any necessary action in response to the missing variable.
Remember, os.environ
simplifies the process of retrieving environment variable values, allowing your Python applications to dynamically adapt to various configurations and environments with ease.
Using third-party libraries to access environment variables in Python
While Python provides built-in methods to access environment variables, there are also several powerful third-party libraries available that can enhance your experience and provide additional features.
Let’s look at one of the three popular examples.
Library 1: Access environment variables using Python-Decouple
Python-Decouple is a versatile library that simplifies the process of managing configuration settings, including environment variables.
It allows you to store configuration values in separate files and provides a convenient interface to access them.
Here’s how to install and use Python-Decouple in your Python app:
Step 1: Install Python-Decouple library
To begin, you need to install Python-Decouple in your Python environment.
Open your terminal or command prompt, activate the virtual environment, and execute the following command:
pip install python-decouple
This command will download and install the Python-Decouple library along with its dependencies, making it available for use in your Python app.
Step 2: Importing the Python Decouple Library
After installing Python-Decouple, you need to import it into your Python script.
Here’s an example:
from decouple import config
In this example, we import the config
function from the decouple
module, which allows us to access environment variables and configuration values using Python-Decouple.
Step 3: Access Environment Variables for your Python App
Now that you have imported Python-Decouple, you can start accessing environment variables in your code.
The config()
function serves as the main entry point for retrieving values.
Here’s an example:
database_url = config('DATABASE_URL')
In this example, config('DATABASE_URL')
retrieves the value associated with the 'DATABASE_URL'
environment variable.
Python-Decouple automatically handles the process of reading the variable from a configuration file, such as .env
, allowing you to keep sensitive information separate from your codebase.
Step 4: Store your Python App Configuration Files in a .env file
Python-Decouple allows you to store your configuration values in separate files, typically in the .env
format.
This practice helps in maintaining a clean separation between your code and sensitive configuration details.
Here’s an example of a .env
file:
First, create a .env file in your app’s folder and add the filename in your .gitignore to avoid committing it in a public repo.
touch .env
In the file, add your app’s configuration files:
DATABASE_URL=postgresql://user:password@localhost:5432/mydatabase
API_KEY=your_api_key_here
Make sure to create a
.env
file in the same directory as your Python script and populate it with your specific environment variable assignments.
To learn more on .env files, why they are used in Python, and how to create them, check out this guide: How to create and use .env files in your Python projects
Step 5: Use Retrieved Environment Values
Once you have retrieved the environment variable values, you can use them in your Python application as needed.
For example:
import psycopg2
# Retrieve the database URL
database_url = config('DATABASE_URL')
# Connect to the database
connection = psycopg2.connect(database_url)
# ...
In this example, we use the retrieved database_url
value to establish a connection to a PostgreSQL database using the psycopg2
library.
The retrieved environment variable allows us to dynamically configure the database connection based on the environment.
By following these steps, you can effectively install and use Python-Decouple in your Python application.
It simplifies the management of environment variables and configuration values, promoting better separation of sensitive information and providing flexibility in different environments.
Library 2: Using Python-Dotenv to retrieve env variables
Python-Dotenv is another popular library that loads environment variables from a .env
file into the os.environ
dictionary.
This approach simplifies the process of setting up and managing your environment variables.
Here’s how to install and use Python-Dotenv in your Python code:
Step 1: Install Python-Dotenv
To get started, you need to install the Python-Dotenv library.
Open your terminal or command prompt and run the following command:
pip install python-dotenv
If you are using a virtual environment for your Python project, activate it first and then install the library.

Step 2: Create a .env
File to Store your Environment Variables
Next, create a .env
file in the root directory of your Python app.
This file will store your environment variables in a key-value format.
Each line should follow the KEY=VALUE
pattern.
For example:
API_KEY=abcdef123456
DATABASE_URL=postgres://user:password@localhost:5432/db_name
Feel free to add as many environment variables as needed, depending on your application’s requirements.
Step 3: Load the Environment Variables
In your Python script, you need to load the environment variables from the .env
file using Python-Dotenv.
Import the dotenv
module and call the load_dotenv()
function.
Here’s an example:
import dotenv
# Load environment variables from the '.env' file
dotenv.load_dotenv()
By calling dotenv.load_dotenv()
, Python-Dotenv reads the contents of the .env
file and loads the environment variables into the os.environ
dictionary.
The dotenv.load_dotenv()
should return True if a .env file exists in the current working directory. If you see a False, you should create the .env file first and inside the Python module directory.

You can now access these variables using os.getenv()
.
Step 4: Access the Environment Variables
Once the environment variables are loaded, you can access them in your Python code using os.getenv()
.
Here’s an example:
import os
# Retrieve the value of the 'DJANGO_SETTINGS_MODULE' environment variable
dj_settings = os.getenv('DJANGO_SETTINGS_MODULE')
# Use the retrieved value
print(dj_settings)
In this example, os.getenv('DJANGO_SETTINGS_MODULE')
retrieves the value of the 'DJANGO_SETTINGS_MODULE'
environment variable. You can assign it to a variable, use it within your code, or perform any necessary operations.

Step 5: Run Your Python App
Ensure that the .env
file is in the same directory as your Python script.
Now, when you run your Python application, Python-Dotenv will automatically load the environment variables from the .env
file, making them accessible throughout your code.
By following these steps, you can seamlessly use Python-Dotenv to load environment variables from a .env
file into your Python application.
This approach simplifies the management of environment-specific configurations and enhances the portability of your code.
Remember to keep the .env
file secure and avoid committing it to version control systems to protect sensitive information.
Library 3: Django-Environ to access Django-specific environment variables
If you are working with Django, the Django-Environ library provides an elegant solution for managing environment variables specific to your Django project.
Django-Environ is a powerful library that simplifies the management of environment variables in your Django applications.
It seamlessly integrates with Django’s configuration system, allowing you to easily access environment variables within your Django settings.
Here’s an example installation and usage of the Django-Environ Library:
Step 1: Install the Django-Environ library
To install Django-Environ, you can use pip
, the Python package installer.
Open your command line interface and execute the following command:
pip install django-environ
This command will download and install Django-Environ along with its dependencies into your Python environment, enabling you to use the library in your Django project.

Step 2: Import Django-Environ and Initialize Environment Configuration
In your Django project, open the settings module (typically settings.py
) and add the necessary import statement to import Django-Environ:
import environ
Next, initialize the Django-Environ configuration by creating an instance of the Env
class.
env = environ.Env(
)
Next, configure your app to take the environment variables from the .env file created in the root directory of your Django application.
Add the following code snippet to your settings module:
environ.Env.read_env(os.path.join(BASE_DIR, '.env'))
This initializes the environment configuration and prepares Django-Environ to manage your environment variables.
Step 3: Access Environment Variables
With Django-Environ configured, you can now access your environment variables within the Django settings.
To retrieve the value of an environment variable, use the env()
method of the env
instance, passing the name of the variable as a string parameter.
Here’s an example:
SECRET_KEY = env('SECRET_KEY')
In this example,
env('SECRET_KEY')
retrieves the value of the'SECRET_KEY'
environment variable and assigns it to theSECRET_KEY
setting.You can replace
'SECRET_KEY'
with the name of the environment variable you want to access.By using
env()
for environment variable retrieval, Django-Environ automatically handles the process of retrieving the value from the underlying environment, whether it’s from an actual environment variable or from a configuration file like.env
.
Step 4: Configuring Environment Variables
To configure your environment variables, you have multiple options with Django-Environ.
One common approach is to use a .env
file.
Create a file named .env
in the root directory of your Django project and define your environment variables with their corresponding values.
Here’s an example:
DEBUG=True
SECRET_KEY=your-django-secret-key
DATABASE_URL=psql://user:[email protected]:8458/database
CACHE_URL=memcache://127.0.0.1:11211
By placing your environment variables in the .env
file, Django-Environ will automatically load them into your Django settings.
Step 5: Using the Environment Variables in Your Django App
Once you have accessed your environment variables in the Django settings, you can utilize them throughout your Django app as needed.
For instance, you might use the retrieved values to configure database connections, API credentials, or other settings specific to your Django project.
# Example usage of environment variables in Django settings
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': env('DATABASE_NAME'),
'USER': env('DATABASE_USER'),
'PASSWORD': env('DATABASE_PASSWORD'),
'HOST': env('DATABASE_HOST'),
'PORT': env('DATABASE_PORT'),
}
}
In this example, the environment variables are used to configure the database connection settings in Django.
By retrieving the values with env()
, you can ensure that your database configuration remains separate from your codebase and easily customizable through environment variables.
How to get environment variables during production
When deploying your Python applications to a production environment, accessing environment variables becomes crucial for proper configuration.
Let’s explore two common scenarios: accessing environment variables in a Platform as a Service (PaaS) such as Heroku and DigitalOcean App Platform, and in a shared hosting live environment.
In a Platform as a Service (PaaS) such as Heroku and Digital Ocean App Platform
PaaS providers like Heroku, AWS Elastic BeanStalk, and DigitalOcean App Platform offer convenient platforms for deploying applications.
They typically provide built-in mechanisms to manage environment variables for your deployed applications.
When creating a new app either in Heroku or Digital Ocean, you have the option to access the app settings tab. In this tab, you should the section for Environment Variables where you can add your environment variables.
To be specific, in Digital Ocean, you can access the environment variable configuration settings by navigating into the Settings tab, then App-Level Environment Variables, and clicking the edit link to add your new environment variables.
Enter the environment variable in the KEYS field and then in the Values field, enter your environment variable value.
For more information, here is the full guide.
Once you have set the environment variables, you can access them within your Python application using os.getenv()
just like with any other deployment scenario.
For example:
import os
# Retrieve the value of the 'API_KEY' environment variable on DigitalOcean App Platform
api_key = os.getenv('API_KEY')
In this example, os.getenv('API_KEY')
retrieves the value of the 'API_KEY'
environment variable on the DigitalOcean App Platform.
One of the best hosting services that you can use for your Python web applications (Django, Flask, etc.) is DigitalOcean. For me, Digital Ocean has been the go-to hosting service whenever I am hosting my web applications and for my clients.
It is easier to configure a new web app, deploy, and scale your website.
The support is great.
And not to forget how affordable their packages are.
To get started with Digital Ocean, use the link below and you will get a discount for your hosting package plus get free credit for testing the platform. If you love it, you can always upgrade.
Link here:
In a shared hosting live environment
In some shared hosting environments, the hosting provider allows you to set environment variables through their cPanel when you are configuring a new Python App.
Creating new environment variables in cPanel using the “Setup New Python App” feature allows developers to provide environment-specific variables for each Python application.
Here is a step-by-step process to set up new environment variables:
Step I: Log in to your cPanel dashboard
Open your web browser and navigate to your cPanel login page.
Enter your credentials to access your cPanel account.
Step II: Locate the “Setup New Python App” Feature
Once logged in, search for the “Setup New Python App” feature.
It may be located under the “Software” or “Applications” section in cPanel.
Click on the “Setup New Python App” icon to proceed.
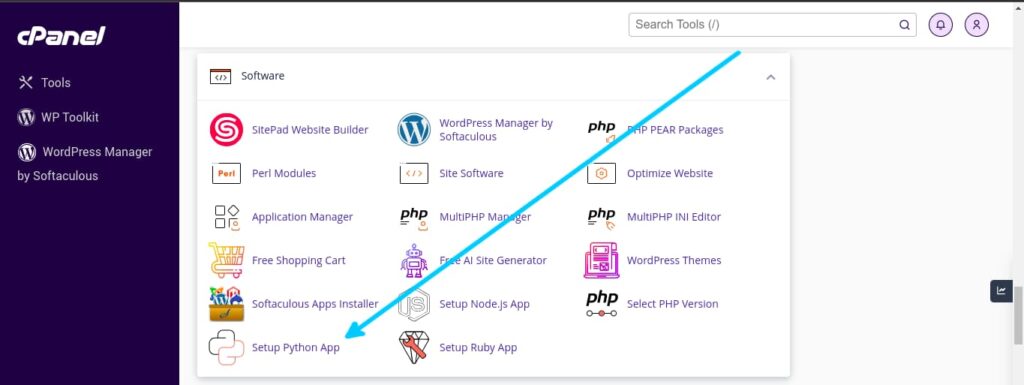
Step III: Select the Python Version and App Directory
Choose the appropriate Python version for your application.
Select the directory where you want to set up your Python application.
If the directory does not exist, you can create a new one or choose an existing one.
Step IV: Provide App Details
Fill in the required information for your Python app, such as the application name, application domain, and application startup file.
Review and confirm the details before proceeding.
Step V: Set Up Environment Variables
In the “Environment Variables” section, you will find a table with two columns: “Variable” and “Value”.
This is where you can add your new environment variables.
- In the “Variable” column, enter the name of your environment variable.
- In the “Value” column, provide the corresponding value for the environment variable.
You can add multiple rows to set up multiple environment variables for your Python app.
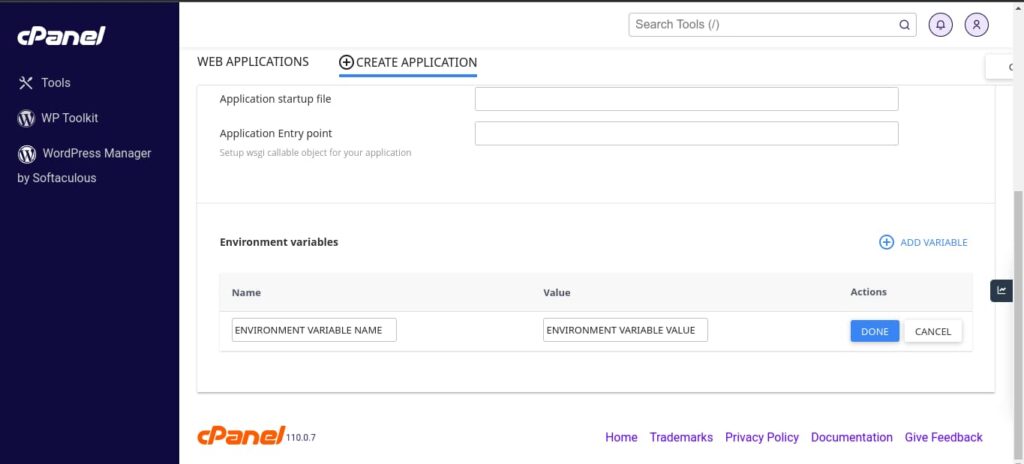
Step VI: Save and Create your Python App
Once you have added all the necessary environment variables, click on the “Create” or “Save” button to create the Python app with the specified environment variables.
cPanel will create the application directory, set up the Python environment, and configure the provided environment variables.
Step VII: Access the Environment Variables in Your Python App
In your Python application code, you can access the environment variables using the os.getenv()
function.
For example:
import os
# Retrieve the value of the 'API_KEY' environment variable
api_key = os.getenv('API_KEY')
By using os.getenv('API_KEY')
, you can access the value of the 'API_KEY'
environment variable within your Python app.
Conclusion
We have explored the intricacies of accessing and utilizing environment variables in Python.
We began by understanding the importance of environment variables in programming, as they allow for dynamic configuration and enhance the flexibility and portability of our applications.
We discussed various methods to access environment variables, including using built-in functions like os.getenv()
and os.environ
, which provides straightforward ways to retrieve specific variables or access all variables as a dictionary-like object.
Additionally, we explored the advantages of leveraging third-party libraries like Python Decouple, Python-Dotenv, and Django-Environ.
These libraries offer advanced features such as configuration file support, type casting, and integration with specific frameworks like Django.
We have looked at different scenarios, including accessing environment variables in local development setups, production environments such as PaaS and shared hosting, and even within cPanel’s “Setup New Python App” feature.
By understanding how to access environment variables, retrieve specific values, handle cases when variables are not set, and utilize third-party libraries, you can enhance the configurability, security, and scalability of your Python applications.
You are now equipped with the expertise and understanding to effectively access and utilize environment variables in Python.
Create, inspire, repeat!
Create, inspire, repeat!