When working with Python scripts, you might have encountered situations where you want to control where the output goes.
Redirecting output is a nifty technique that allows you to send the output of your .py script to a file, the console, or even manipulate it in other ways.
In this article, we will dive into the world of output redirection in Python and explore how you can effortlessly steer your script’s output wherever you desire.
Let’s start by understanding what output redirection means when working with Python programs.
What does redirecting output mean in Python?
Output redirection simply means changing the destination of your script’s output.
By default, when you run a Python script, the output is displayed on the console (also known as standard output).
However, with output redirection, you can direct the output to other places like a file, another program, or even capture it for further manipulation within your script.
You may ask,
Why is output redirection useful in Python?
Output redirection can be immensely helpful in various scenarios.
Imagine you have a lengthy script that generates a large amount of output.
Instead of scrolling through all the text on the console, you can redirect it to a file, making it easier to analyze and share the results.
Additionally, output redirection allows you to automate tasks by sending the output of one script as input to another, enhancing the flexibility and efficiency of your programs.
That’s not all:
- Data Analysis and Reporting: Redirecting output to a file enables easy data analysis and reporting. By capturing the output in a file, you can efficiently process and analyze large amounts of data, generate insightful reports, and extract valuable information.
- Debugging and Troubleshooting: Redirecting output to a file or console is immensely helpful for debugging and troubleshooting purposes. It allows you to inspect the program’s output, error messages, and any unexpected behavior, aiding in identifying and resolving issues efficiently.
- Log File Management: Redirecting output to a log file is an essential practice for proper log file management. It allows you to consolidate program logs in one place, making it easier to monitor and analyze the program’s execution, track errors, and monitor system behavior.
- Automation and Scripting: Output redirection plays a vital role in automating tasks and scripting workflows. By redirecting output, you can seamlessly integrate multiple scripts or programs, allowing one script’s output to serve as the input for another, automating complex workflows, and enhancing overall productivity.
- Batch Processing: In scenarios where you need to process a large number of files or execute repetitive tasks, output redirection proves invaluable. By redirecting output, you can handle batch processing effectively, ensuring that the output is captured and organized efficiently.
- Collaboration and Sharing Results: Redirecting output to a file facilitates collaboration and sharing of results with others. It allows you to capture the program’s output in a readable format, making it easier to share results, communicate findings, and collaborate with colleagues or clients.
- User-Friendly Interfaces: Redirecting output to the console or a graphical user interface (GUI) enhances the user experience. It allows users to view relevant information, progress updates, and error messages in real-time, providing a more interactive and informative interface.
- Unit Testing and Quality Assurance: Redirecting output aids in unit testing and quality assurance processes. By capturing the output, you can compare the expected results with the actual output, ensuring that the program functions as intended and meets the desired quality standards.
- System Monitoring and Logging: Redirecting output to a log file is crucial for system monitoring and logging. It enables the continuous capture of system events, error messages, and relevant information, providing insights into system performance, security, and overall stability.
- Customization and Flexibility: Output redirection offers customization and flexibility options to suit specific requirements. By redirecting output, you can tailor the destination of the output to meet your needs, whether it’s a file, another program, a network socket, or a variable for further manipulation.
All these become useful when:
- Capturing Program Output: Redirecting output to a file allows you to capture and store the output of your Python program. This is particularly helpful when running scripts that produce a large amount of output, such as data processing or simulation programs. By redirecting the output to a file, you can review it at your own pace, extract relevant information, and ensure nothing important is missed.
- Error and Debugging Information: When troubleshooting your code, it’s crucial to have easy access to error messages and debugging information. By redirecting the output to a console or log file, you can conveniently view any error messages, stack traces, or print statements that aid in identifying and fixing issues within your code.
- Creating Log Files: Output redirection is commonly used to generate log files that track the execution of a program. By redirecting output to a log file, you can record important events, timestamps, and specific actions taken during the program’s execution. Log files are invaluable for debugging, auditing, and analyzing program behavior over time.
- Automation and Scripting Workflows: When automating tasks or creating complex scripting workflows, output redirection is instrumental in managing and processing output efficiently. By redirecting output to different files or programs, you can seamlessly chain multiple scripts together, enabling one script to consume the output of another as input. This allows you to automate complex workflows, perform data transformations, or trigger subsequent actions based on specific output conditions.
- Generating Reports and Documentation: Redirecting output to a file enables you to generate reports and documentation directly from your Python scripts. By capturing the output in a structured format, you can extract relevant information, format it appropriately, and create reports that provide insights, summaries, or detailed documentation of your program’s behavior and results.
- System Monitoring and Alerts: Output redirection plays a vital role in system monitoring and generating alerts. By redirecting output to a monitoring system or tool, you can track system metrics, capture error conditions, and trigger notifications or alerts based on specific output patterns or thresholds. This allows you to proactively monitor the health and performance of your systems and take necessary actions when issues arise.
By leveraging output redirection in these common scenarios, you can gain more control over your program’s output, streamline workflows, simplify debugging, automate tasks, and enhance the overall user experience.
It’s a powerful technique that empowers you to effectively manage and utilize the valuable output generated by your Python scripts.
With that, let’s see how you can redirect simple to complex output in Python.
How to redirect output in Python
There are two major approaches to redirecting output in Python:
- File redirection and
- Redirecting output to standard streams.
Each approach offers distinct advantages and is suited for different use cases.
In this section, we will explore both approaches and provide step-by-step instructions on how to redirect output in Python using each method.
Whether you want to capture the output in a file for later analysis or redirect it to standard streams for immediate viewing, this guide will equip you with the knowledge and techniques to efficiently redirect output in your Python scripts.
So, let’s dive in and discover how you can take control of your program’s output in Python!
Redirecting Python script output to a file
When working with Python scripts, you might have noticed that the output usually appears on your screen.
But what if you want to save that output to a file instead?
That’s where redirecting output to a file comes in handy.
Output redirection allows you to capture and store the output of your Python script in a file of your choice.
It’s like having a virtual secretary who diligently writes down everything your script outputs.
Why is file redirection important, you ask?
Well, let me tell you.
Redirecting output to a file can be incredibly useful in many situations.
Imagine you have a script that generates a lot of data, logs, or error messages.
Instead of having them flood your terminal or command prompt, you can neatly collect them in a file for later analysis.
It not only keeps your screen clutter-free but also allows you to review and study the output at your own pace.
Plus, it provides a record of your script’s execution that you can refer back to whenever needed.
The applications of redirecting output to a file are diverse and powerful. Here are a few use cases:
- Data Analysis: When you’re dealing with large datasets, redirecting output to a file allows you to save the results for further analysis, visualization, or sharing with others. It’s like capturing the essence of your script’s findings in a convenient and portable format.
- Logging and Debugging: During the development process, it’s crucial to keep track of errors, warnings, and debug information. By redirecting output to a file, you can create detailed logs that help you identify and fix issues more effectively.
- Automated Tasks and Batch Processing: If you have scripts running automatically or as part of a larger batch process, redirecting output to a file ensures that you have a record of their execution. This can be particularly helpful when you want to monitor the progress, track any errors, or review the results later on.
Well, file redirection provides all these advantages, but,
How do I redirect output to a file with an example code?
Step-by-step guide on redirecting output to a file
Here is a walk-through process on how to perform file redirection in Python:
Step 1: Open a File for Writing
To redirect the output to a file, you first need to create and open a file in write mode.
You can use the built-in open()
function in Python for this purpose.
Let’s say we want to redirect the output to a file called “output.txt”.
Here’s how you can open the file:
output_file = open("output.txt", "w")
Step 2: Use the sys
Module to Redirect Output
Python provides the sys
module, which allows you to access system-specific parameters and functions.
We can use it to redirect the output to the file we opened in the previous step.
To do this, you need to import the sys
module at the beginning of your script:
import sys
Step 3: Write Redirected Output to the File
Now that you have opened the file and imported the sys
module, it’s time to actually redirect the output.
In Python, the standard output is represented by the stdout
attribute of the sys
module.
By assigning the opened file to sys.stdout
, we redirect the output to that file.
Here’s how you can do it:
sys.stdout = output_file
Step 4: Run Your Script and Observe the Output
With the output redirection in place, run your script as you normally would.
Any print statements or other output commands will now write to the file “output.txt” instead of appearing on the console.
This allows you to capture and save the output for later use.
Step 5: Close the File
Once you are done redirecting the output and no longer need to write to the file, it’s essential to close it properly.
This ensures that all the data is saved and resources are freed up.
You can close the file using the close()
method:
output_file.close()
Real-world examples and illustrations of file redirection in action
Let’s consider a real-world example to understand how redirecting output to a file can be beneficial.
Imagine you are working on a weather analysis script in Python.
This script fetches weather data from an API, performs calculations, and provides useful information to the user.
In this scenario, you want to redirect the script’s output to a file called “weather_report.txt” so that you can generate a report containing the analyzed weather data.
Here’s how you can create a Python script that redirects output to a file:
import sys
def fetch_weather_data():
# Simulating fetching weather data from an API
return {
"temperature": 25,
"humidity": 70,
"wind_speed": 15
}
def generate_weather_report(weather_data):
# Simulating generating a weather report
report = f"Weather Report:\n\nTemperature: {weather_data['temperature']}°C\nHumidity: {weather_data['humidity']}%\nWind Speed: {weather_data['wind_speed']} km/h"
return report
def redirect_output_to_file(output_file_path):
# Open the file for writing
output_file = open(output_file_path, "w")
# Redirect output to the file
sys.stdout = output_file
# Fetch weather data from API
weather_data = fetch_weather_data()
# Perform calculations and generate the report
report = generate_weather_report(weather_data)
# Print the report
print(report)
# Close the file
output_file.close()
# Demonstrate the usage
output_file_path = "weather_report.txt"
redirect_output_to_file(output_file_path)
In this code, we first define two functions: fetch_weather_data()
and generate_weather_report()
.
These functions simulate fetching weather data from an API and generating a weather report, respectively.
The redirect_output_to_file()
function takes an output_file_path
parameter and performs the steps we discussed earlier.
It opens the file specified by output_file_path
in write mode, redirects the output to the file using sys.stdout
, fetches weather data, generates the weather report, and prints it.
Finally, the file is closed.
To demonstrate the usage, we set the output_file_path
variable to “weather_report.txt” and call the redirect_output_to_file()
function.
The output will be redirected to the file “weather_report.txt”.
If I execute the code:
python weather_report.py
Then open the output .txt file, I should see the results of the program showing the weather today.
cat weather_report.txt
# results
Weather Report:
Temperature: 25°C
Humidity: 70%
Wind Speed: 15 km/h
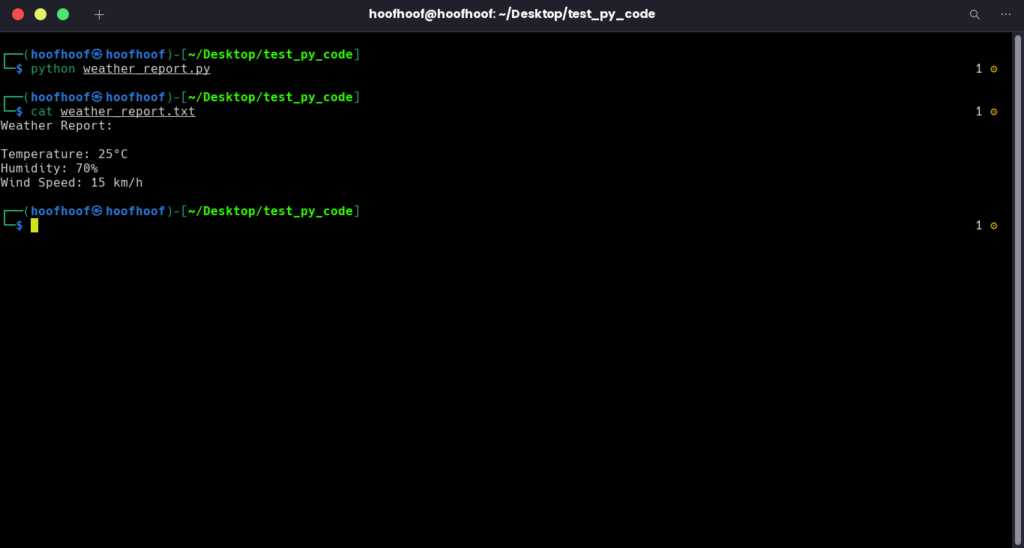
By redirecting the output to a file, you have a detailed weather report stored in “weather_report.txt”.
You can review the report later, share it with others, or even analyze the data further.
Redirecting the output in this manner helps you separate the computational logic from the presentation of results.
It allows you to focus on the analysis while keeping the output organized and easily accessible.
Alright, now that it is clear.
How do I redirect the output of a print statement to a file, instead of showing it to the terminal?
How to redirect print output to a file in Python
There is another approach to redirecting output in Python, specifically targeting print statements.
By utilizing this method, you can directly redirect the output of print statements to a file of your choice.
This can be particularly useful when you want to isolate and capture specific output for analysis or documentation purposes.
Let’s see how.
To redirect print output to a file, we can leverage the power of the print()
function itself, along with file object handling.
By specifying the file
parameter in the print()
function, we can direct the output to a file instead of the standard output (console).
Let’s delve into the steps involved in redirecting print output to a file.
Step 1: Open the File for Writing
Begin by opening the file in write mode, just as we did when redirecting output in the previous section.
You can use the open()
function to create and open the desired file, specifying the file name and the mode as “w” for writing.
output_file = open("print_output.txt", "w")
Step 2: Redirect the Print Output to the File
To redirect the output of print statements to the file, pass the output_file
object as the value for the file
parameter of the print()
function.
Any subsequent print statements will then write their output directly to the specified file.
print("Hello, world!", file=output_file)
Step 3: Close the File
After you have finished redirecting the print output and no longer need to write to the file, it’s crucial to close the file using the close()
method.
This ensures that all the data is saved and resources are freed up.
output_file.close()
Real-World Example:
Let’s consider a real-world example to illustrate the practical usage of redirecting print output to a file.
Suppose you are developing a data processing script that performs complex calculations on large datasets.
During the execution, the script prints intermediate results for debugging and monitoring purposes.
However, printing these large datasets to the console might be overwhelming and hinder readability.
By redirecting the print output to a file, such as “data_processing_log.txt”, you can capture and analyze the intermediate results more efficiently.
This allows you to review the printed data later or share it with other team members for collaborative debugging.
Here’s an example of redirecting print output to a file in the context of our data processing script:
output_file = open("data_processing_log.txt", "w") # Redirect print output to the file print("Starting data processing...", file=output_file) # Perform complex calculations on the dataset # ... intermediate_result = "Some very complex calculations producing large amount of data" # Print intermediate results to the file print("Intermediate result:", intermediate_result, file=output_file) # Continue data processing # ... output_file.close()
In the example above, the script starts by opening the file “data_processing_log.txt” in write mode.
It then uses the
print()
function with thefile
parameter to redirect print output to the file.Intermediate results are printed directly to the file, ensuring a cleaner and more organized debugging process.
By incorporating this approach, you can have a focused and structured log of your program’s print output, making it easier to trace the execution flow and identify potential issues during data processing.
Alternatively, you can use shell redirection to redirect the output of a print statement to a file.
Here’s how:
How to redirect print output to a file using shell redirection (>)
There is an alternative way to redirect print output to a file in Python that involves utilizing the shell redirection operator (>
).
This method allows you to redirect the output of an entire script, including all print statements, to a file from the command line.
Let’s explore:
Step 1: Run the Script with Shell Redirection Operator
To redirect the print output of a Python script to a file using the shell redirection operator, you need to execute the script from the terminal with the appropriate redirection syntax.
In the command line or the Terminal, use the following format:
python script.py > output.txt
This command runs the script script.py
and redirects its output to the file output.txt
.
The >
operator tells the shell to send the output to the specified file instead of the console.
Step 2: Observe the Redirected Output
Once the script is executed with the shell redirection operator, the output generated by print statements will be written to the specified file (output.txt
in this example).
You can open the file using a text editor or read it programmatically to examine the redirected output.
The alternative approach of using the shell redirection operator offers a convenient way to redirect the entire output of a Python script, including print statements, to a file.
It simplifies the process by allowing you to specify the redirection directly in the command line or the terminal, without modifying the script itself.
Note: Keep in mind that this approach redirects the entire output of the script, including any errors or warnings, to the file.
It may be useful for capturing all output in a single file, but it does not provide fine-grained control over individual print statements.
Files redirection is relatable, but are there other ways to redirect Python script output?
Yes, indeed!
In addition to redirecting output to files, Python provides a powerful mechanism for redirecting script output using standard streams.
How to redirect Python script output using standard streams
By utilizing the standard streams—sys.stdin
, sys.stdout
, and sys.stderr
—you can dynamically redirect input, output, and error streams to different destinations, such as files or even other streams.
This gives you fine-grained control over where the script’s output goes and provides flexibility in handling input and error messages.
In this section, we will explore how to redirect script output using the standard streams, empowering you with greater control and customization.
But before that,
What are standard streams in Python?
In simple terms, standard streams are the primary channels through which a program communicates with the outside world, specifically for input, output, and error messages.
They include:
- Standard Input (
sys.stdin
): This stream is responsible for receiving input data into a Python script. By default, it reads input from the keyboard, allowing users to interact with the program. However, we can redirect it to other sources, such as files or even other programs, to automate input or read data from different sources. - Standard Output (
sys.stdout
): This stream is used for displaying output from a Python script. By default, it sends output to the console, making it visible to the user. However, we can redirect it to different destinations, such as files or other programs, to capture or redirect the script’s output. - Standard Error (
sys.stderr
): This stream is responsible for printing error messages and other diagnostic information. Similar to standard output, it is typically displayed on the console by default. However, we can also redirect it to different destinations, such as error logs or files, for better error handling and analysis.
Understanding the concept of standard streams is essential as it forms the foundation for redirecting Python script output.
By redirecting these streams, you can control where the input comes from, where the output goes, and how error messages are handled.
So, in this case, to redirect a Python script output, you will need the stdout stream for the purpose of this article. stderr should be left to do what it does best, which is to report error message logs.
With that said, let’s see
How to redirect output to standard streams in Python
Step 1: Import the sys Module
To work with standard streams, you need to import the sys
module in your Python script.
This module provides access to the standard streams and allows you to interact with them programmatically.
import sys
Step 2: Redirect Output to a Different Destination
To redirect the output to a different destination, such as a file or the console, you can assign a new value to sys.stdout
.
Here’s an example:
sys.stdout = open("output.txt", "w")
In the example above, the output is redirected to a file named “output.txt”.
You can replace the file name with any other file you want to use as the destination for the output.
Alternatively, you can assign
sys.stdout
tosys.__stdout__
to restore the default behavior and send the output to the console.
Step 3: Perform Script Execution
After redirecting the output, you can proceed with the execution of your Python script as usual.
Any print statements or output commands within the script will now send the output to the destination you specified.
Real-World Example:
Let’s consider a real-world example to demonstrate the practical usage of redirecting output to standard streams.
Imagine you are developing a script to calculate and display various statistics based on a dataset.
As the script executes, you want to display the statistics on the console for real-time monitoring and simultaneously save a log file containing the output for future analysis.
To achieve this, you can redirect the output of the script to both the console and a log file.
Here’s an example of how you can implement this:
import sys def calculate_statistics(): # Filler data dataset = [10, 15, 8, 12, 6] # Calculate statistics mean = sum(dataset) / len(dataset) minimum = min(dataset) maximum = max(dataset) # Return the calculated statistics return mean, minimum, maximum # Redirect output to a log file log_file = open("script_log.txt", "w") sys.stdout = log_file # Perform calculations and generate statistics statistics = calculate_statistics() # Print statistics to the console print("Statistics:") print("Mean:", statistics[0]) print("Minimum:", statistics[1]) print("Maximum:", statistics[2]) # Close the log file log_file.close()
In the example above, we start by importing the
sys
module.We then open a log file named “script_log.txt” in write mode and assign it to
sys.stdout
.This redirects the output to the log file.
Next, we perform calculations and generate statistics.
The
print()
statements are used to display the statistics on the console in a readable format.After executing the script, the log file will contain the entire output, including the displayed statistics.
This allows you to monitor the script’s progress on the console while having a comprehensive log for further analysis.
By redirecting output to standard streams, you can tailor the destination of your script’s output to meet specific requirements.
Whether it’s displaying output on the console or redirecting it to a file, this approach provides flexibility and control over where the output goes.
With such control, can we redirect output to multiple streams, like:
Redirect output to a file and screen?
Using the Python logging
module is another approach to redirecting output to both a file and the screen simultaneously.
The logging
module provides a flexible way to handle logging and allows us to direct log messages to different destinations, such as files and the console.
Let’s explore how to redirect output to a file and the screen using the logging
module.
1. Import the logging
module
import logging
2. Configure the logging settings
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
handlers=[
logging.FileHandler('script_log.txt'), # Output to a file
logging.StreamHandler() # Output to the console
]
)
In this example, we configure the logging settings using basicConfig()
.
We set the logging level to INFO
to capture messages of severity INFO
and above.
The format
parameter specifies the format of the log messages.
The handlers
list contains two handlers: FileHandler
to output to a file and StreamHandler
to output to the console.
3. Log messages using the logging methods
logging.info("This message will be logged to both the file and the screen")
Use the logging methods (info()
, warning()
, error()
, etc.) to log messages.
These messages will be directed to both the file specified in the FileHandler
and the console (screen) due to the configured handlers.
4. Close the logging handlers (optional)
logging.shutdown()
If you want to ensure all log records are flushed and the logging system is properly shut down, you can call logging.shutdown()
.
By following these steps, you can redirect output to both a file and the screen simultaneously using the logging
module.
Here’s a complete example showcasing the above steps:
import logging
# Configure the logging settings
logging.basicConfig(
level=logging.INFO,
format='%(asctime)s - %(levelname)s - %(message)s',
handlers=[
logging.FileHandler('script_log.txt'), # Output to a file
logging.StreamHandler() # Output to the console
]
)
# Log messages
logging.info("This message will be logged to both the file and the screen")
# Close the logging handlers (optional)
logging.shutdown()
When you run this script, the log message “This message will be logged to both the file and the screen” will be simultaneously displayed on the console and saved to the specified log file (script_log.txt
).
Using the logging
module provides you with greater flexibility in configuring logging settings, formatting log messages, and directing output to multiple destinations.
It’s a powerful tool for handling logging requirements in Python programs.
But, Steve, where can a Python programmer use logging in a real-world case?
Well, Django or Flask web applications deployed on a Linux server are good examples.
For this example, let’s consider Django:
Here’s a full guide showing how a Django application can be deployed on a Linux server e.g VPS server with logging capabilities:
1. Set up a Linux server (e.g., Ubuntu) and connect to it via SSH.
2. Install Python and other necessary packages:
sudo apt update
sudo apt install python3 python3-pip python3-venv
3. Create a virtual environment for your Django project:
python3 -m venv myenv
source myenv/bin/activate
4. Install Django:
pip install django
5. Create a new Django project:
django-admin startproject myproject
6. Change into the project directory:
cd myproject
7. Run the Django development server:
python manage.py runserver 0.0.0.0:8000
This starts the development server on port 8000, allowing external connections.
8. Open a web browser and enter the server’s IP address or domain name followed by :8000
. You should see the default Django welcome page.
To implement logging and see the errors Django is reporting both on the Terminal window and to a file, you can implement logging like this:
9. Open the settings.py
file in your Django project:
nano myproject/settings.py
10. Add the following lines at the top of the file to import the logging
module:
import logging
11. Locate the LOGGING
section in the settings.py
file, and replace it with the following configuration:
LOGGING = {
'version': 1,
'disable_existing_loggers': False,
'handlers': {
'console': {
'class': 'logging.StreamHandler',
},
'file': {
'class': 'logging.FileHandler',
'filename': '/var/log/django/myproject.log', # Replace with desired log file path
},
},
'root': {
'handlers': ['console', 'file'],
'level': 'INFO', # Set desired logging level
},
}
This configuration sets up two log handlers: console
for logging into the console and file for logging into a file.
The log file path is specified in the 'filename'
parameter.
12. Save the settings.py
file and exit the editor.
13. Open the views.py
file in your Django project:
nano myproject/views.py
14. Add the following import statement at the top of the file to import the logger:
import logging
15. In the view function, add logging statements as needed.
For example:
def my_view(request):
logger = logging.getLogger(__name__)
logger.info('This is an info message')
logger.warning('This is a warning message')
logger.error('This is an error message')
# Your view logic here...
These logging statements will log messages to both the console and the specified log file.
16. Save the views.py
file and exit the editor.
17. Restart your Django development server:
python manage.py runserver 0.0.0.0:8000
18. Interact with your Django application, and the log messages will be recorded in the log file /var/log/django/myproject.log
(or the specified path).
By following these steps, you have configured logging in your Django project.
You can place logging statements in various parts of your code to capture important information, warnings, and errors.
This allows you to monitor the behavior of your Django application and troubleshoot any issues that may arise.
The log file can be accessed and analyzed to gain insights and track the application’s activities.
FAQs
Can I redirect only error messages without affecting regular output?
You can redirect only error messages without affecting regular output in Python. Separate the stdout to use it for logging regular output and stderr streams for logging error messages. You can do this by using the subprocess.PIPE and communicate() method. Capture and write the output and error messages to different files.
Can I redirect output to a network socket instead of a file?
You can redirect output to a network socket instead of a file in Python. By leveraging the socket library, you can establish a connection to a specific network address and send the output to that socket.
Here’s how you do that:
Import the necessary modules:
import sys
import socket
Set up the socket connection:
server_address = (‘localhost’, 1234) # Replace with the desired server address and port
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect(server_address)
Redirect the output to the socket:
sys.stdout = sock.makefile(‘w’)
This line redirects the standard output to the socket by creating a file-like object from the socket connection.
Print statements or any output will now be sent to the network socket:
print(“Hello, World!”)
print(“This is a test.”)
The output of these print statements will be sent to the network socket established in Step 2.
Restore the original output stream:
sys.stdout = sys.__stdout__
This line restores the original standard output.
Close the socket connection:
sock.close()
It’s important to close the socket connection when you’re finished sending the output.
By following these steps, you can redirect the output of your Python script to a network socket instead of a file. This can be useful when you want to send the output to a remote server or another application for real-time processing or monitoring.