The terminal provides a command-line interface that allows you to interact with Python’s powerful features, execute code snippets on-the-fly, and run entire scripts effortlessly.
In this article, we will explore various techniques and methods to execute Python code and .py files in the terminal, equipping you with the knowledge and skills to leverage this invaluable tool in your Python development workflow.
Let’s dive right in!
How to execute Python code in the Terminal
Executing Python code directly in the terminal is an essential skill that every beginner Python programmer should learn.
It allows you to run your Python programs and see their results right away.
We will explore two simple methods of executing Python code in the terminal:
- Using the Python Interactive Shell (REPL) and
- Executing Python code directly.
These methods will help you quickly test your code, understand how it works, and become more comfortable with running Python programs.
So, let’s dive in and learn how to use the terminal to run your Python code with ease.
1. Using the Python Interactive Shell (REPL) to run Python code
The Python Interactive Shell, also known as the REPL (Read-Eval-Print Loop), is a handy tool that lets you write and run Python code directly in the terminal.
It’s a fantastic way to experiment with Python, test small snippets of code, and get immediate feedback on your commands.
So, how do we use the Python Interactive Shell effectively to execute Python code?
Here’s the step-by-step process:
Step 1: Open the Terminal
Look for the program on your computer that lets you type in commands.
It might be called “Terminal,” “Command Prompt,” or “Shell.”
On Linux, the app should be the Terminal. Alternatively, you may press CTRL + ALT + T simultaneously to launch the Terminal app.
It should look like this, once you launch it:
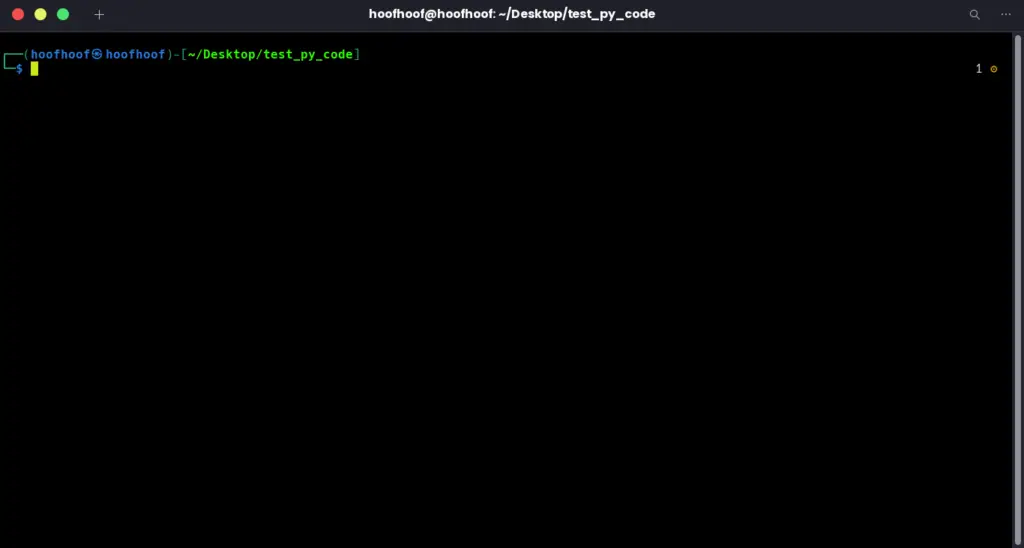
Step 2: Start the Python Interactive Shell by typing python
or python3
and hitting Enter
Type python
or python3
in the terminal and hit Enter.
You should see a prompt that looks like >>>
, indicating that you are now in the Python Interactive Shell.
See the image below:
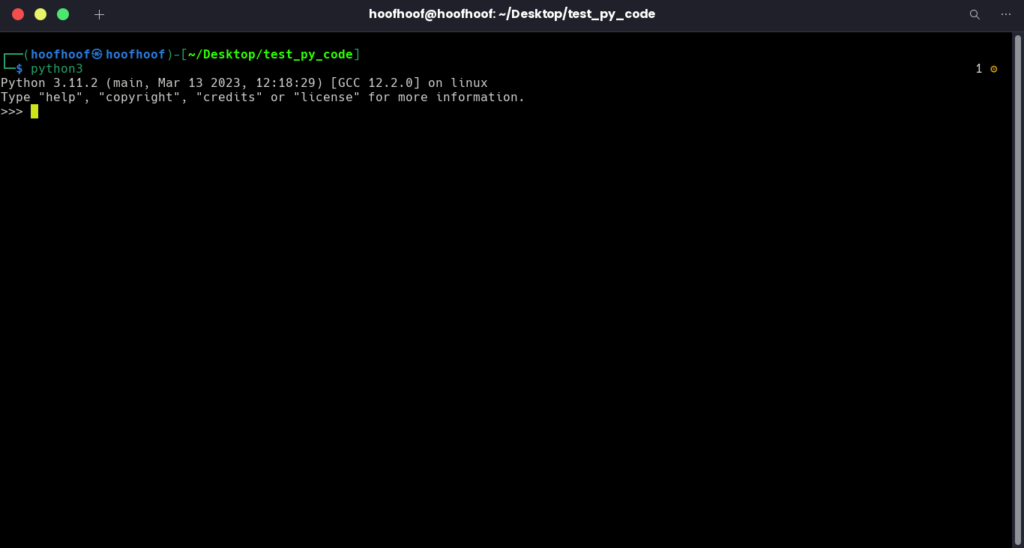
Step 3: Write and execute Python code in the shell
You can now start writing Python code directly in the shell.
Type a line of code, such as print("Hello, world!")
, and hit Enter.
The shell will immediately execute the code and display the output if there is any.
This is a very simple example.
How can you execute Python code spanning multiple lines of code, especially with tabs such as for loop?
Well, here’s how you do it.
Let’s say, for example, you want to execute a simple for loop or an if condition.
Here’s how you can do it:
When writing Python code that involves tabs, such as for loops or if conditions, it’s important to format the code correctly to avoid syntax errors.
Here’s how you can insert tabs without encountering syntax errors in the Python Interactive Shell:
1. Start by typing the initial line of code that requires indentation, such as a for loop or an if condition.
For example, let’s say you want to write a for loop that prints numbers from 1 to 5:
for i in range(1, 6):
2. After typing the initial line, press the Tab key on your keyboard. This will insert an appropriate indentation (typically equivalent to four spaces) to align the following lines of code under the for loop.
for i in range(1, 6): # The cursor is automatically indented here
3. Now, you can continue writing the remaining code that should be executed within the loop, maintaining the indentation level.
For example, let’s print the numbers using the
print()
function:for i in range(1, 6): print("Number:", i)
By indenting the subsequent lines of code, you ensure that they are part of the for loop and will be executed iteratively.
4. After completing the code block that requires indentation, you can press Enter to execute the code.
The Python Interactive Shell will run the code and display the output, if any, based on the indentation.
Number: 1 Number: 2 Number: 3 Number: 4 Number: 5
Remember that consistent and proper indentation is crucial in Python. The use of tabs or spaces for indentation should remain consistent throughout your code, even when using the interactive shell.
Step 4: Get help or information about a particular Python command or function
If you’re unsure about how a particular Python command or function works, you can ask for help directly in the shell.
Simply type help(topic)
and replace topic
with the specific command or function you want to learn more about.
The Python Interactive Shell provides useful documentation and guidance to assist you in your learning journey.
Let’s say you want to understand how the
len()
function works, which is used to determine the length of a string or a list.Here’s how you can obtain help and information about this function in the Python Interactive Shell:
1. Type
help(len)
in the shell and press Enter.The
help()
function is a built-in Python function that displays information and documentation about the specified object or function.help(len)
2. After executing the command, the Python Interactive Shell will provide detailed information about the
len()
function.It will explain what the function does, what arguments it expects, and what it returns.
Help on built-in function len in module builtins: len(obj, /) Return the number of items in a container.
The displayed information will give you insights into how to use the
len()
function effectively.3. You can scroll through the help documentation using the arrow keys or the Page Up and Page Down keys on your keyboard to explore more information about the function.
It may include examples, explanations of optional parameters, and related functions or concepts.
4. Once you have finished reviewing the documentation, you can exit the help viewer by pressing the Q key on your keyboard.
Using the help()
function in the Python Interactive Shell allows you to quickly access information and understand how different Python commands and functions work.
It’s a valuable resource to clarify any doubts or uncertainties you may have about specific Python features, enabling you to write more effective and accurate code.
Step 5: Exit the Python Interactive Shell
To exit the Python Interactive Shell, type exit()
or quit()
and hit Enter.
You will return to the regular terminal prompt.
By using the Python Interactive Shell, you can quickly test ideas, understand Python concepts, and gain confidence in your programming skills.
It’s a valuable tool for beginners and experienced programmers alike, enabling you to experiment and iterate on your code in a hassle-free manner.
Next, let’s see how you can execute Python code directly from the terminal without having to access the Python Interactive Shell.
2. Executing Python code directly in the terminal using python -c
In addition to using the Python Interactive Shell, you can also execute Python code directly in the terminal.
Sometimes, you may want to execute a quick Python command or code snippet without creating a separate .py file or accessing the Python Interactive Shell.
The python -c
command allows you to achieve this by directly executing Python code in the terminal.
Here’s how you can do it:
Open your terminal or command prompt
Opening the Terminal by locating the app or pressing CTRL + ALT + T.
Type python -c
followed by your Python code enclosed in double quotes.
For example: python -c "print('Hello, World!')"
.
python -c "print('Hello, World!')"
Press Enter to execute the command, and you’ll see the output in the terminal.
With our hello world example, here are the results:
$ python -c "print('Hello, World!')"
Hello, World!
What are the benefits of using python -c to execute Python code?
- Quick execution: It enables you to execute Python code without the need for a separate script or accessing the interactive shell.
- One-liner convenience: You can write concise Python commands or code snippets within a single line.
- Useful for simple tasks: It’s handy for performing quick calculations, printing results, or running short Python expressions.
But Steve, you forgot something: how can I execute a multiline Python code, such as a for loop, using the python -c
command.
Well,
Executing a multiline code, such as a for loop, using the python -c
command can be achieved by properly formatting the code within the command string.
Here’s an example of how you can execute a multiline code, like a for loop, using the python -c
command:
- Open your terminal or command prompt.
- Type
python -c
followed by your Python code enclosed in triple quotes ("""
). - Inside the triple quotes, write your multiline Python code, such as a for loop.
- Press Enter to execute the command, and you’ll see the output in the terminal.
For example:
Let’s say you want to execute a simple for loop that prints numbers from 1 to 5.
Here’s how you can do it using python -c
:
python -c """
for i in range(1, 6):
print(i)
"""
If you are using Python3:
python3 -c """
for i in range(1, 6):
print(i)
"""
In the above example, the code block for the for loop is enclosed within triple quotes ("""
).
This allows you to write multiline Python code within a single command.
Each line of code within the triple quotes is treated as a separate line of Python code.
When you execute the python -c
command, the Python interpreter will interpret the multiline code and execute it accordingly.
In this case, it will execute the for loop and print numbers from 1 to 5 as output.
Using this approach, you can execute more complex multiline code, including loops, conditionals, and other Python constructs, directly in the terminal using the python -c
command.
That’s it for the section of executing Python code in the Terminal.
How about a script?
You know, a .py file.
It’s actually easier!
How to run Python script (.py) in the Linux Terminal
Running Python scripts in the Linux terminal is a fundamental skill for any Python programmer. It allows you to effortlessly execute your Python programs and witness their magic unfold.
Whether you’re a beginner or a seasoned developer, understanding how to run Python scripts directly in the terminal is a valuable skill that will empower you to bring your ideas to life.
Let’s see how
Method 1: Running Python scripts in the terminal using python
interpreter
To run Python scripts in the terminal, we’ll use the Python interpreter.
This method allows you to execute larger chunks of code saved in .py files.
Follow these steps to get started:
Create your Python script
- Open a text editor and write your Python code.
- Save the file with a .py extension. For example, save it as “my_script.py”.
Here’s a simple command to create a my_script.py file using the Terminal and nano.
touch my_script.py
Write your Python code
Open a text editor and write some Python code.
Let’s start with a fun joke generator example:
# Example: A Joke Generator
import random
jokes = [
"Why don't scientists trust atoms? Because they make up everything!",
"Why don't skeletons fight each other? They don't have the guts!",
"Why did the scarecrow win an award? Because he was outstanding in his field!",
"What do you call a fish wearing a crown? King mackerel!",
"Why did the bicycle fall over? Because it was two-tired!"
]
joke = random.choice(jokes)
print(joke)
Save this code in our file named “my_script.py”. Feel free to modify or come up with your own code if you want.
Open the terminal
Launch the terminal or command prompt on your operating system.
Navigate to the script’s directory
Use the “cd” command to navigate to the directory where your Python script is saved.
For example, if your script is saved in the “Documents” folder, type:
cd Documents
Execute the script
In the terminal, enter the following command to run your Python script:
python3 my_script.py
Note: If you have multiple versions of Python installed, specify the version by using “python3” instead of “python” if necessary.
View the output and enjoy the lame joke
Press Enter to execute the command.
You’ll see the output of your Python script displayed in the terminal.
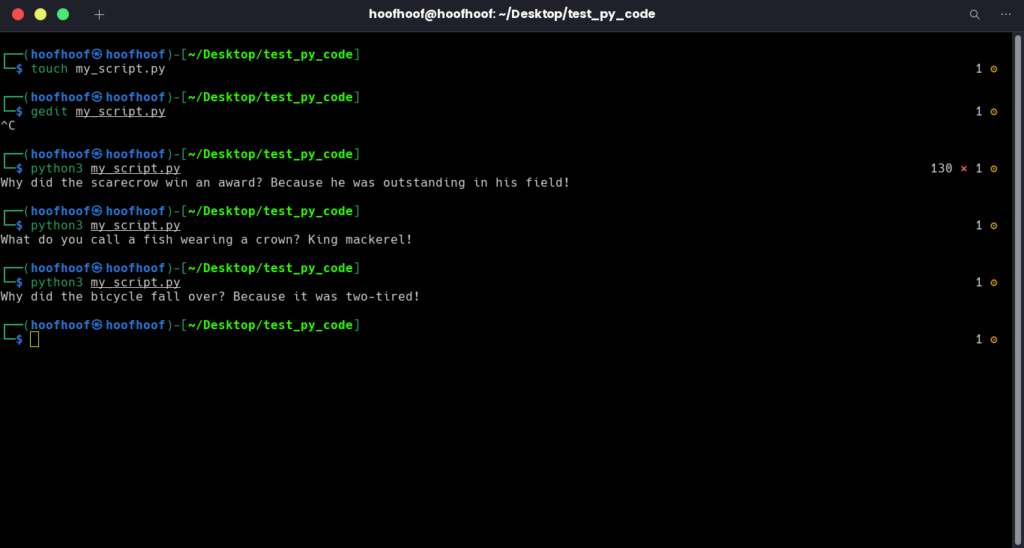
By following these steps, you can easily run your Python scripts in the terminal using the Python interpreter.
This method is useful when you have longer scripts or when you want to automate tasks.
Remember to navigate to the script’s directory before executing it in the terminal.
Method 2: Using shebang line to execute Python scripts directly
Another method for executing Python scripts directly in the terminal is by using the shebang line.
This approach allows you to run your Python scripts without explicitly invoking the Python interpreter.
By adding a simple line of code at the beginning of your script, you can make it executable and run it as a standalone program.
Let’s explore how to use the shebang line effectively to execute Python scripts directly in the terminal.
Step 1: Create a new Python script or open an existing one in a text editor
As an example, I will create a script called, dad_jokes.py
. You can create a Python script with any name you can think of.
For me, I want to create a random dad joke generator Python program to run on my phone and crack me up when I am in that boring late afternoon lecture class.
So,
Create the file:
touch dad_jokes.py
Step 2: At the very top of your script, insert the following line of code
#!/usr/bin/env python
This line is known as the shebang line and tells the system to use the Python interpreter to execute the script.
Write the rest of the code according to the intended functionality of the script. For me, I will retrieve some random dad jokes like this:
#!/usr/bin/env python
import requests
def fetch_dad_joke():
response = requests.get("https://icanhazdadjoke.com/", headers={"Accept": "text/plain"})
return response.text
def main():
joke = fetch_dad_joke()
print(joke)
if __name__ == "__main__":
main()
Step 3: Save your script with a .py extension, such as “my_script.py”.
Step 4: Make your script executable by running the following command in the terminal
chmod +x dad_jokes.py
The chmod +x command grants execution permission to the script.
Navigate to the directory containing your Python script in the terminal when executing the command above.
Step 5: Execute the Python script using ./script_name.py
To execute our example script, type the following command:
./dad_jokes.py
Replace “dad_jokes.py” with the actual name of your script.
Press Enter to run the script, and the output will be displayed in the terminal.
By using the shebang line method, you can make your Python scripts more self-contained and easily executable with a single command, improving the overall convenience and user experience.
Note: The shebang line may vary depending on the location of the Python interpreter on your system.
The example above assumes a standard location.
If your Python interpreter is installed in a different location, adjust the shebang line accordingly.
How to run Python scripts with command-line arguments
In many cases, you may want to provide inputs or customize the behavior of your Python scripts without modifying the code itself.
This is where command-line arguments come into play.
By allowing you to pass values or flags through the terminal when executing a script, command-line arguments offer a flexible and dynamic way to interact with your Python programs.
In this section, we will cover the syntax for passing arguments, accessing them within the script, provide examples, and discuss the benefits and use cases of this powerful feature.
By mastering the art of utilizing command-line arguments, you’ll be able to create more versatile and user-friendly Python scripts that adapt to different scenarios and user inputs.
Let’s see how.
Step 1: Define your script’s functionality and the expected command-line arguments
Before diving into the implementation, you need to have a clear understanding of what your script does and what arguments it expects from the command line.
Define the purpose and functionality of your script, and determine which values or options need to be passed as command-line arguments.
Example:
Let’s consider a simple example where we want to calculate the sum of two numbers provided as command-line arguments to our Python script.
Step 2: Import the sys
module in your Python script to access the command-line arguments
To access the command-line arguments within your script, you need to import the sys
module, which provides access to system-specific parameters and functions.
Here’s an example:
import sys
Step 3: Access the command-line arguments using sys.argv
, which returns a list of strings representing the arguments
The sys.argv
variable contains the command-line arguments passed to your script.
It is a list of strings, where the first element (sys.argv[0]
) represents the script’s name, and subsequent elements contain the arguments.
arguments = sys.argv[1:] # Exclude the script name from the list of arguments
Step 4: Analyze and process the command-line arguments within your script to perform the desired actions or modifications
Once you have accessed the command-line arguments, you can analyze and process them based on your script’s logic.
Convert the arguments to the appropriate data types and perform any necessary validations or transformations.
Example:
if len(arguments) != 2:
print("Please provide exactly two numbers as command-line arguments.")
return
num1 = int(arguments[0])
num2 = int(arguments[1])
Step 5: Run your Python script in the terminal, providing the necessary command-line arguments
To execute your script with command-line arguments, you need to run it in the terminal or command prompt.
Provide the required arguments separated by spaces after the script’s name.
Example:
python sum_calculator.py 5 10
Step 6: Retrieve and utilize the passed arguments within your script’s logic.
Once the script is executed with command-line arguments, you can retrieve the passed values and utilize them within your script’s logic to perform the desired operations.
Example:
result = calculate_sum(num1, num2)
print("The sum is:", result)
So your final Python script should look like this:
import sys
def calculate_sum(num1, num2):
return num1 + num2
def main():
arguments = sys.argv[1:] # Exclude the script name from the list of arguments
if len(arguments) != 2:
print("Please provide exactly two numbers as command-line arguments.")
return
num1 = int(arguments[0])
num2 = int(arguments[1])
result = calculate_sum(num1, num2)
print("The sum is:", result)
if __name__ == "__main__":
main()
Benefits and Use Cases of running Python scripts with command-line arguments
Customization
Command-line arguments allow users to customize script behavior without modifying the code.
For example, you can pass filenames, parameters, or flags to control different aspects of the program’s execution.
Example:
import sys
def process_file(filename):
# Code to process the file
print("Processing file:", filename)
def main():
if len(sys.argv) != 2:
print("Please provide a filename as a command-line argument.")
return
filename = sys.argv[1]
process_file(filename)
if __name__ == "__main__":
main()
In this example, the script expects a filename as a command-line argument.
The process_file()
function is responsible for processing the file specified in the argument.
Users can execute the script with a specific filename, like python my_script.py data.csv
, where data.csv
is the file they want to process.
Automation
By utilizing command-line arguments, you can automate repetitive tasks or batch processing.
This allows you to write scripts that can handle multiple inputs or perform operations on a large set of data.
Example:
import sys
import os
def process_files(directory):
for filename in os.listdir(directory):
# Code to process each file
print("Processing file:", filename)
def main():
if len(sys.argv) != 2:
print("Please provide a directory as a command-line argument.")
return
directory = sys.argv[1]
process_files(directory)
if __name__ == "__main__":
main()
In this example, the script expects a directory as a command-line argument.
The process_files()
function iterates through all the files in the specified directory and performs some processing on each file.
Users can execute the script with a directory path, like python my_script.py /path/to/files
, where /path/to/files
is the directory containing the files they want to process.
Interoperability
Command-line arguments make it easier to integrate Python scripts into larger systems or workflows, enabling seamless communication between different programs or scripts.
Example:
import sys
import database_module
def generate_report(database, report_type):
# Code to generate the report based on the database and report type
print("Generating report:", report_type)
def main():
if len(sys.argv) != 3:
print("Please provide the database and report type as command-line arguments.")
return
database = sys.argv[1]
report_type = sys.argv[2]
generate_report(database, report_type)
if __name__ == "__main__":
main()
In this example, the script expects the database and report type as command-line arguments.
The generate_report()
function generates a report based on the specified database and report type.
Other scripts or systems can invoke this script and provide the necessary arguments, such as python my_script.py production_database summary
, where production_database
is the database name and summary
is the report type.
You can customize, automate, and integrate your Python scripts into various workflows by utilizing command-line arguments, making them more versatile and powerful tools.
FAQs
Can I run Python scripts from Python interactive shell?
No, you cannot directly run a Python script from the Python interactive shell. The interactive shell is primarily used for interactive exploration and execution of Python code, while running scripts requires executing them from the command line or an integrated development environment (IDE).
Conclusion
You have learned the various methods and techniques for running Python code and .py files in the terminal. Besides, practiced how to execute Python code directly in the terminal and execute Python scripts with command-line arguments.
These skills are valuable for programmers as they offer flexibility, customization, and automation options when working with Python scripts.
By mastering these techniques, you can enhance your productivity.
Remember to practice and experiment with different approaches to further expand your knowledge and proficiency in running Python code and scripts from the terminal.
Happy coding!