When working with Python, variables are a fundamental concept that every programmer must understand.
Variables are used to store values that can be accessed and manipulated throughout a program.
While variables are typically assigned a name that is relevant to their purpose, it is not uncommon for a programmer to realize that a variable needs to be renamed after it has been declared.
This could be due to a change in requirements or simply a desire to improve the readability of the code.
However, the question arises –
Can you rename a variable in Python?
You can rename a variable in Python. Renaming a variable can be useful for improving code readability, or when there is a need to update the name of a variable for reasons that helps curate the relevancy of the variable to the value it stores.
There are several approaches to renaming a variable in Python. One way to do it is to assign the value of the old variable to a new variable with the desired name. For instance, if you have a variable named old_variable
, you can create a new variable named new_variable
and assign the value of old_variable
to it.
Another approach is to use tuple unpacking, where you create a new variable and assign it the value of the old variable using the syntax new_variable, = old_variable,
.
You can also use the setattr()
function to set the value of a new variable with the desired name and delete the old variable.
Additionally, manually highlighting a variable name or using the “Find and Replace” feature in Python code editors and integrated development environments (IDEs) is another approach to renaming a variable in Python.
But first,
Why do we rename variables in Python?
There are several scenarios where a programmer may need to rename a variable in Python. Here are some of the most common ones:
- The variable name is not descriptive or meaningful: Programmers often start writing code with quick variable names, but as they progress and the codebase becomes more complex, it becomes necessary to have more meaningful and descriptive variable names.
- The variable name conflicts with a reserved keyword or built-in function: In Python, there are certain keywords and built-in functions that cannot be used as variable names. If a variable has been named after a keyword or built-in function, it may need to be renamed to avoid conflicts and errors.
- The variable name needs to be updated to reflect changes in requirements: As the requirements of a program change, it may become necessary to update the variable names to reflect these changes.
- The variable name is too long or difficult to type: If a variable name is too long or difficult to type, it can be beneficial to rename it to a shorter, more manageable name.
- The variable name does not follow naming conventions: Python has naming conventions that should be followed to make code more readable and consistent. If a variable name does not follow these conventions, it may need to be renamed.
- The variable name is used inconsistently throughout the codebase: If a variable is used inconsistently throughout the codebase, it can be confusing for other programmers who are working on the same code. Renaming the variable to a more consistent name can improve code readability and maintainability.
- The variable name is misspelled or contains errors: If a variable name is misspelled or contains errors, it can cause errors and make the code hard to read. Renaming the variable to the correct name can resolve these issues.
With that in mind, let’s see each approach you can take to rename variables in Python.
How to rename a variable in Python
Renaming a variable in Python can be a necessary step in making code more readable and maintainable. Fortunately, there are several ways to accomplish this task in Python.
In this section, we will explore the different approaches that can be used to rename a variable in Python, including assigning the value of the old variable to a new variable with the desired name, using tuple unpacking, and using the setattr()
function to set the value of a new variable with the desired name and delete the old variable.
Rename a variable by assigning it a new variable name
This approach is the simplest but effective approach to renaming variables in Python. This approach involves assigning the value of the old variable to a new variable with a different name, effectively renaming the variable.
For example, suppose we have a variable x
that stores a person’s name, and we want to rename it to person_name
.
We can do this by creating a new variable person_name
and assigning the value of x
to it:
x = "Steve"
person_name = x
In this example, the value of the old variable x
is assigned to the new variable person_name
, effectively renaming the variable.
While this approach may not be the most elegant or efficient way to rename variables, it is a simple and reliable method that can be used in many situations.
It is important to note, however, that reusing variable names can make the code harder to understand, especially if the variable is used for different purposes in different parts of the code.
It’s generally better to use descriptive variable names that reflect the purpose of the variable to make the code more readable and maintainable.
Using the as
keyword to rename a variable
Using the as
keyword to rename a variable is a straightforward approach that works well for simple cases. The as
keyword allows you to create an alias for a variable, which can then be used in place of the original variable name.
Another useful application of the as
keyword is to rename long import names. When importing modules or specific objects from modules, the names can sometimes be long and cumbersome to use repeatedly. In these cases, using the as
keyword to provide a shorter, more convenient name can improve code readability and efficiency.
For example, consider the following code:
import pandas as pd
data = pd.read_csv('my_data.csv')
Here, we import the popular data analysis library pandas
and use the as
keyword to create an alias pd
for the module name. This allows us to use the shorter name pd
in place of pandas
throughout the code.
Similarly, we can use the as
keyword to rename specific objects from a module:
from sklearn.linear_model import LinearRegression as LR
model = LR()
In this example, we import the LinearRegression
class from the sklearn
module and create an alias LR
for the class name.
We can then use the shorter name LR
in place of LinearRegression
when creating an instance of the class.
Overall, using the as
keyword to rename long import names can make the code more concise and easier to read.
However, it’s important to use aliases that are meaningful and do not obscure the original name’s purpose.
Use tuple unpacking to rename a Python variable
Another approach to renaming a variable in Python is to use tuple unpacking.
This involves assigning the old variable name to a tuple with a new name, and then unpacking the tuple into the new variable name.
For example, suppose we have a variable x
that we want to rename to y
. We can do this using tuple unpacking as follows:
x = 5
(x, y) = (x, x)
print(y) # Output: 5
In this example, we first assign the value 5
to the variable x
.
We then create a tuple (x, y)
where the first element is the old variable name x
and the second element is the new variable name y
.
Finally, we unpack the tuple into the new variable name y
, effectively renaming x
to y
.
We can confirm that the value of y
is 5
by printing it to the console.
This approach to renaming variables can be useful when we need to rename multiple variables simultaneously, or when we want to swap the values of two variables.
Let’s say we have three variables,
x
,y
, andz
, and we want to rename them toa
,b
, andc
respectively.We can use tuple unpacking to rename all three variables at once, like this:
x, y, z = 1, 2, 3 a, b, c = x, y, z print(a, b, c) # Output: 1 2 3 # Swap the values of a and b a, b = b, a print(a, b, c) # Output: 2 1 3
In this example, we first assign the values
1
,2
, and3
to the variablesx
,y
, andz
.We then use tuple unpacking to rename the variables to
a
,b
, andc
.Finally, we swap the values of
a
andb
using tuple unpacking again.This demonstrates how tuple unpacking can be used to rename multiple variables simultaneously and to swap the values of two variables.
However, it’s important to note that using tuple unpacking can make the code harder to read if the variables being renamed are not closely related. Additionally, as with any approach to renaming variables, it’s important to test the code thoroughly after making the changes to ensure that it still works correctly.
Use the dict
method pop
to rename a variable
The dict method pop
can be used to rename a variable in Python by removing the old variable name from a dictionary and assigning the value to a new variable with a different name.
This approach is useful when the variable is stored in a dictionary and needs to be renamed while keeping its value intact.
Here’s an example:
# Create a dictionary with a variable named 'x'
my_dict = {'x': 5}
# Rename the variable 'x' to 'y' using the dict method pop
y = my_dict.pop('x')
# Print the value of 'y'
print(y) # Output: 5
In this example, the dictionary my_dict
contains a variable named x
with the value 5
.
The pop
method is used to remove the key-value pair with the key x
from the dictionary, and the value 5
is returned.
The value is then assigned to a new variable named y
, effectively renaming the variable from x
to y
.
While this approach can be useful in some situations, it should be used with caution as it modifies the original data structure and can lead to unexpected behavior if the dictionary is used elsewhere in the code.
Here’s an example to illustrate how using pop method to rename a dictionary variable can lead to KeyError in cases where you may need to access the original key value.
# Create a dictionary with a variable named 'x' my_dict = {'x': 5} # Rename the variable 'x' to 'y' using the dict method pop y = my_dict.pop('x') # Use the original dictionary elsewhere in the code print(my_dict) # Output: {} # Attempt to access the removed variable print(my_dict['x']) # Raises a KeyError: 'x'
In this example, the dictionary
my_dict
contains a variable namedx
with the value5
. Thepop
method is used to remove the key-value pair with the keyx
from the dictionary, and the value5
is returned.The value is then assigned to a new variable named
y
, effectively renaming the variable fromx
toy
.However, if the original dictionary is used elsewhere in the code, the removal of the
x
key can lead to unexpected behavior.In this example, the code attempts to access the removed variable
x
from the dictionarymy_dict
, which raises aKeyError
because the key no longer exists in the dictionary.This is an example of why using the
pop
method to rename variables should be used with caution, as it can modify the original data structure and lead to unexpected behavior if the data structure is used elsewhere in the code.
Additionally, it may not be the most efficient approach for renaming variables that are not stored in a dictionary.
Use the globals()
or locals()
function to rename a variable
Using the globals()
or locals()
function in Python can be another way to rename a variable, although it is not recommended and can be risky.
These functions allow you to access and modify the global or local namespace, respectively, which includes all the variables defined in your program.
However, modifying the namespace directly can lead to unintended consequences and make the code harder to understand and maintain.
Here’s an example of how to use the globals()
function to rename a variable:
x = 5
print(x) # Output: 5
globals()['x'] = 10
print(x) # Output: 10
In this example, the globals()
function is used to access the global namespace, and the value of the x
variable is changed from 5
to 10
.
The print()
function is then used to verify that the value of x
has been updated.
While this approach may work in some cases, it is generally not recommended because it can lead to unexpected behavior and make the code harder to understand and debug.
Instead, it’s better to use one of the safer and more straightforward approaches to renaming variables in Python, such as using renaming the variable completely in all its instances.
Use the setattr()
function to set the value of a new variable
The setattr()
function can be used to dynamically set the value of a new variable in Python. This can be useful when renaming variables, as it allows you to create a new variable with a new name and assign the old variable’s value to it.
For example, suppose you have a program that uses a variable named num_items
, but you want to rename it to total_items
.
You can create a new variable total_items
and assign num_items
‘s value to it using setattr()
:
class ShoppingCart:
def __init__(self):
self.num_items = 10
setattr(self, 'total_items', self.num_items)
def print_items(self):
print("Number of items:", self.num_items)
print("Total number of items:", self.total_items)
cart = ShoppingCart()
cart.print_items()
In this example, the setattr()
function is used to create a new variable named total_items
and assign the value of num_items
to it.
Now you can use total_items
in your code instead of num_items
, and the value will be the same.
It’s worth noting that
setattr()
can also be used to modify the value of an existing variable, as well as to create new variables.However, using
setattr()
to modify existing variables can be error-prone and can lead to unexpected behavior, so it’s generally better to use other methods such as assignment or tuple unpacking to rename variables.
Manually highlight a variable name and rename it
Manually highlighting a variable name and renaming it is a simple and straightforward approach to renaming a variable in Python.
This method involves selecting the variable name using the cursor and typing in the new name.
However, this method is only suitable for small programs with a few variables, as it can become tedious and error-prone for larger programs with many variables.
For example, suppose we have a program that calculates the area of a rectangle based on its length and width, with the variables length
and width
.
If we decide to rename the variable length
to l
, we can manually highlight the variable name and rename it as follows:
# Calculate the area of a rectangle
width = 5
length = 10
area = width * length
print("The area of the rectangle is:", area)
# Rename the variable "length" to "l"
l = 10
area = width * l
print("The area of the rectangle is:", area)
In this example, we manually highlighted the variable name length
and renamed it to l
in the second part of the program.
However, as the program grows larger and more complex, manually renaming variables can become difficult and error-prone, which is why other approaches such as using the Find and Replace or tuple unpacking may be more suitable.
Use the “Find and Replace” feature in Python IDEs/code editors to rename variables
Another way to rename variables in Python is to use the “Find and Replace” feature in Python IDEs or code editors.
This feature allows you to search for all occurrences of a variable name in your code and replace them with a new name in one go, making the renaming process quicker and more efficient.
For example, let’s say we have a Python script that contains the variable my_var
throughout the code, and we want to rename it to new_var
.
We can use the “Find and Replace” feature in our code editor to do this:
- Open the Python script in your code editor.
- Press “Ctrl+F” (or “Cmd+F” on Mac) to open the “Find” dialog box.
- In the “Find” field, type in the old variable name,
my_var
. - In the “Replace” field, type in the new variable name,
new_var
. - Click “Replace All” to replace all occurrences of
my_var
withnew_var
in the code.
After using the “Find and Replace” feature, all instances of my_var
in the code will be replaced with new_var
.
However, it’s important to be cautious when using this feature, as it can also unintentionally replace other words or names that contain the same characters as the variable name.
So, it’s always a good practice to review the code carefully after using the “Find and Replace” feature to ensure that the changes have been made correctly and that the code still works as expected.
Let’s see how you can use Find and Replace feature on some of the most common IDEs and code editors.
How to change all variable names in Python vs code
To change all variable names in Python using VSCode, you can follow these steps:
1. Open the project folder in VSCode.
2. Use the Find and Replace function to find all occurrences of the old variable name
To do this, use the shortcut Ctrl+Shift+H
on Windows or Linux or Cmd+Shift+H
on Mac, or go to the “Edit” menu and select “Find in Files”.
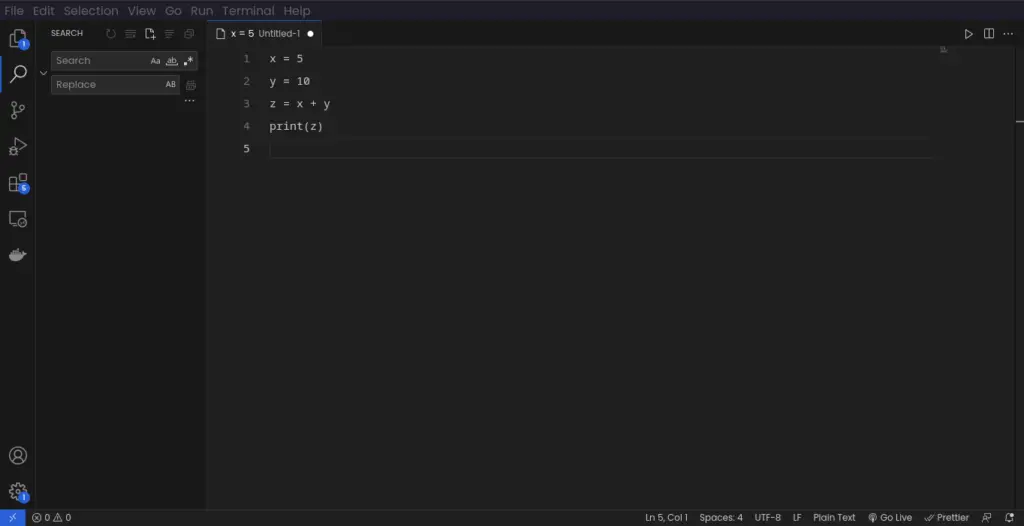
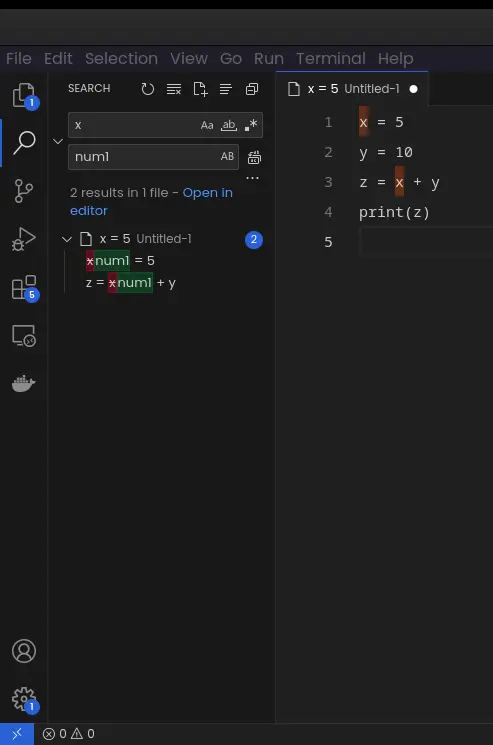
In the “Find” field, enter the old variable name. In the “Replace” field, enter the new variable name. Then, click “Replace All”.
VSCode will prompt you to confirm the changes before making them. Review the changes to ensure that the old variable names are being replaced with the correct new variable names.
And voila!
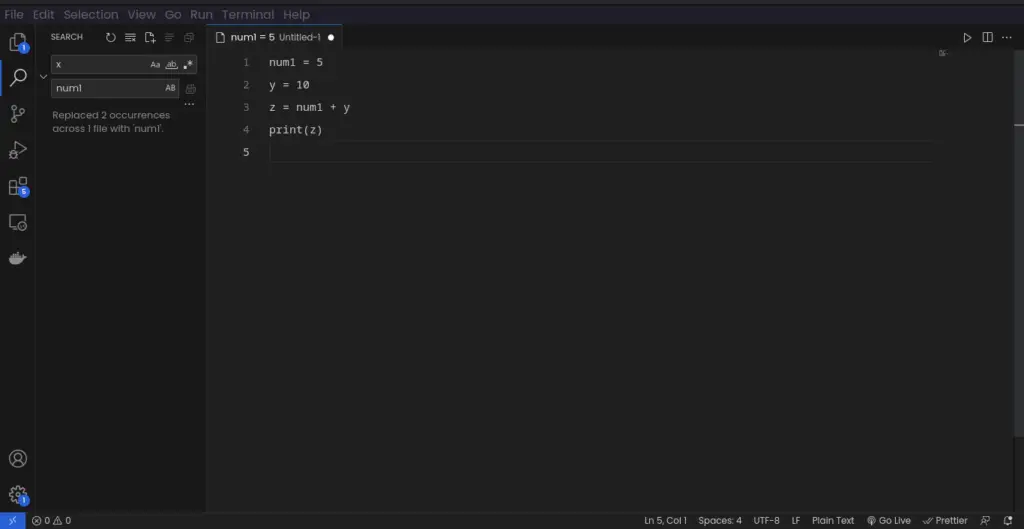
If you have multiple files in your project, VSCode will prompt you to confirm changes for each file. Confirm each change to complete the renaming process.
By following these steps, we can easily rename all occurrences of a variable name in our Python code using VSCode.
How to change all Python variable names in Pycharm IDE
In PyCharm IDE, you can change all Python variable names using the “Rename” feature.
This feature allows you to rename all instances of a variable in a project, making it easy to update variable names throughout the codebase.
To change all variable names in PyCharm, you can follow these steps:
Step 1: Highlight and Right-click on the variable name you want to change
To change the name of this variable to new_var
using PyCharm, you can highlight and right-click on the variable name in the IDE.
Step 2: Click on the “Refactor” option in the context menu or press CTRL/CMD + F6
Select “Refactor” > “Rename” or press CTRL + F6 on Windows or Linux. On Mac, press CMD + F6
Step 3: Click on the “Rename” option in the submenu
Step 4: Enter the new name for the variable and press Enter
Enter the new name for your variable, in this case, new_var
Step 5: Review the changes and click on “Do Refractor” to apply renaming changes
Click “Do Refactor” and let Pycharm do the work. PyCharm will then update all instances of my_var
to new_var
throughout the project.
Be sure to check and test your code works correctly after renaming your variable.
How to change all variable names in spyder
In Spyder, you can change all variable names in your code by using the Find and Replace feature.
Here are the steps to rename variables in Spyder:
- Open the file that you want to modify in Spyder.
- Click on the “Search” icon in the toolbar, or press “Ctrl + R” on your keyboard to open the “Find and Replace” dialog box.
- In the “Find” field, enter the name of the variable that you want to rename.
- In the “Replace with” field, enter the new name for the variable.
- Click on the “Replace All” button to replace all occurrences of the old variable name with the new name.
For example, suppose you have a Python script that defines two variables, x
and y
, and you want to rename them to width
and height
, respectively.
Here is how you can do it in Spyder:
Open the Python script in Spyder
x = 20
y = 12
Press “Ctrl + R” to open the “Find and Replace” dialog box.
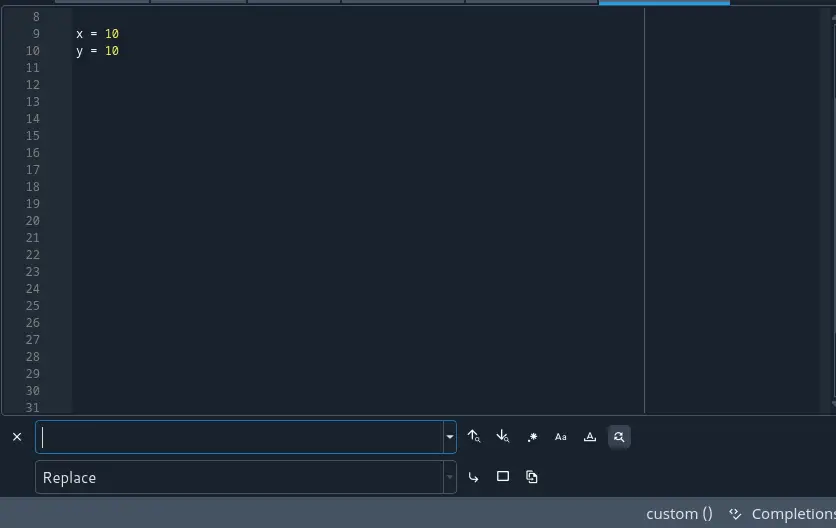
In the “Find” field, enter “x”.
In the “Replace with” field, enter “width”.
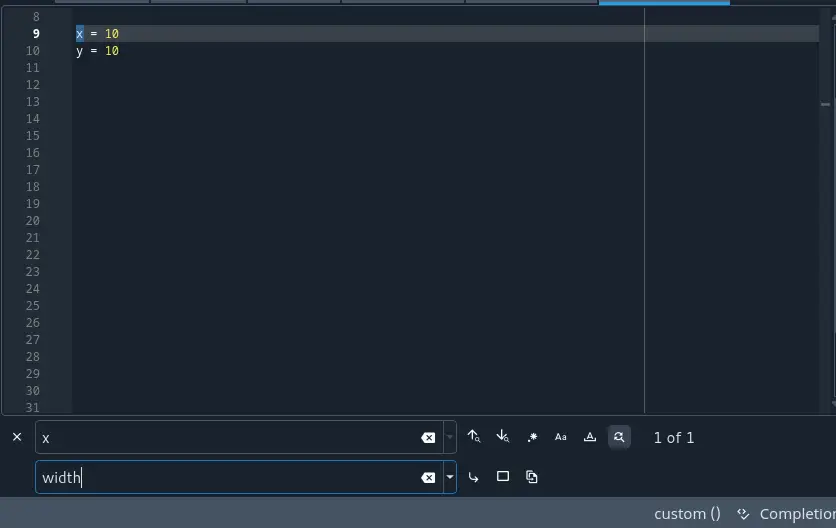
Click on the “Replace All” button to replace all occurrences of “x” with “width”.
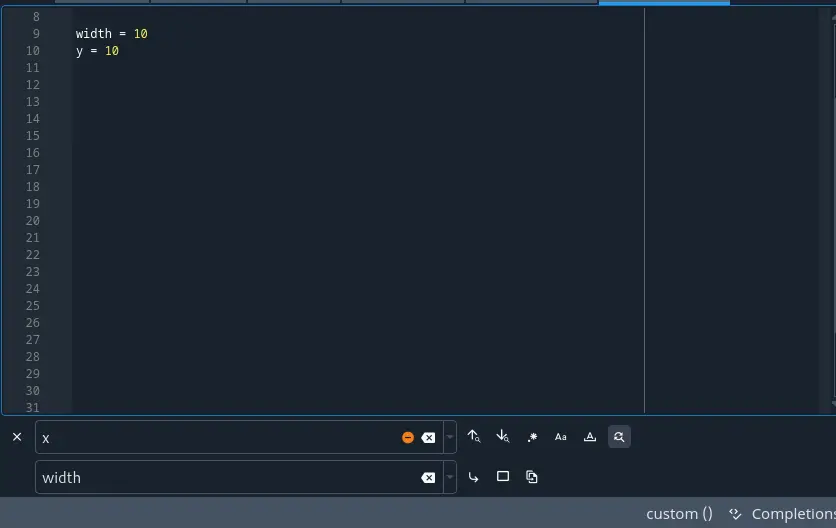
Repeat steps 3-5, but this time enter “y” in the “Find” field and “height” in the “Replace with” field.
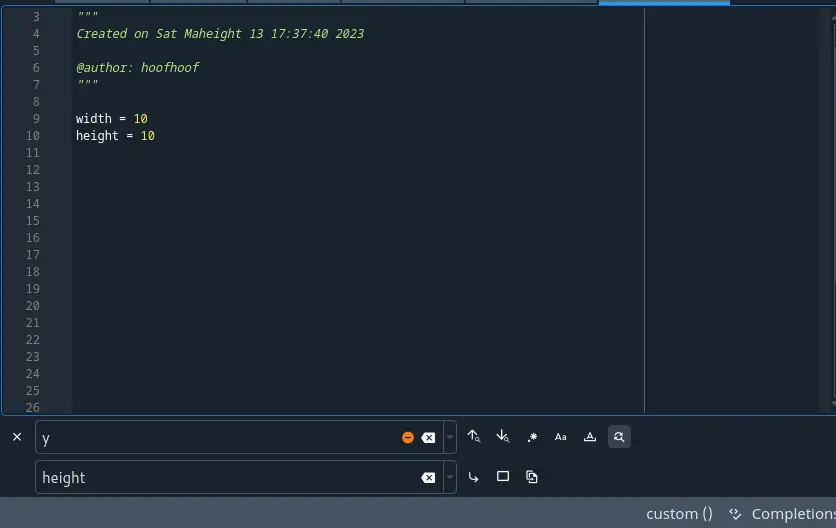
Common pitfalls to avoid when renaming Python variables
Renaming variables in Python can be a useful technique, but it’s important to avoid common pitfalls that can arise.
Here are some of the most common pitfalls to avoid when renaming Python variables:
- Not updating all references to the variable: One of the most common pitfalls when renaming variables is forgetting to update all the references to the variable in the code. This can cause bugs and errors that are hard to track down. It’s essential to do a thorough search of the codebase and update all references to the variable.
- Changing the variable type: When renaming a variable, it’s important to ensure that the new variable has the same type as the old variable. If the new variable has a different type, it can cause errors and bugs in the code.
- Forgetting to update function arguments: If the variable being renamed is used as an argument in a function, it’s essential to update the function signature to reflect the new variable name. Failing to do so can cause errors when the function is called.
- Renaming a variable that is used in a library or API: If a variable that is being renamed is used in a library or API, it can cause issues when the library or API is updated. It’s important to understand the impact of renaming a variable in a library or API before making any changes.
- Using “Find and Replace” indiscriminately: While using the “Find and Replace” feature in code editors can be a quick way to rename variables, it can also introduce errors if used indiscriminately. It’s essential to review all changes made by “Find and Replace” to ensure that the correct variables have been renamed.
By avoiding these common pitfalls, as a programmer, you can ensure that the process of renaming variables in Python is smooth and error-free.
Best practices for renaming variables in Python
Renaming variables in Python can improve code readability and maintainability.
Here are some best practices to follow when renaming variables in Python:
- Use descriptive variable names: Choose variable names that clearly indicate what the variable represents to avoid changing it in the future. This can make it easier to understand the code and reduce the need for renaming variables in the future.
- Rename variables in small steps: Rather than renaming all instances of a variable at once, rename them in small steps. This can help avoid errors and make it easier to track changes.
- Test the code after renaming: After renaming variables, it’s important to test the code to ensure that it works as intended. This can help catch any errors that may have been introduced during the renaming process.
- Update all references to the variable: Ensure that all references to the variable have been updated to the new name. This can help avoid errors and make the code easier to understand.
- Document the changes: It’s important to document any changes made to the codebase, including the renaming of variables. This can help other developers understand the changes and reduce the likelihood of confusion.
- Use a code editor or IDE that supports renaming variables: Many code editors and IDEs have built-in support for renaming variables. Using these tools can make the renaming process faster and more efficient.
FAQs
Can a variable be changed during execution?
A variable can be changed during execution in Python. In fact, this is one of the fundamental features of Python and other programming languages – the ability to modify and update variables as the program runs.
For example, consider the following code:
x = 10
print(x) # Output: 10
x = 20
print(x) # Output: 20
In this code, the variable x
is assigned the value 10, which is then printed to the console.
The value of x
is then changed to 20, and the updated value is printed to the console.
This shows that variables in Python can be modified and updated as the program runs.
It’s worth noting that not all variables in Python can be modified during execution.
For example, variables declared as constants using the const
keyword in Python 3.8+ cannot be modified once they are assigned a value.
Similarly, variables declared with the final
keyword in Python 3.10+ cannot be reassigned to a new value after they are initialized.
Can you reuse variable names in Python?
Yes, you can reuse variable names in Python. In Python, a variable is just a name that refers to a value stored in memory, and the value can be changed at any time. When you assign a new value to a variable that already has a value, the old value is replaced with the new value.
For example, consider the following code:
x = 5
print(x) # Output: 5
x = "Hello"
print(x) # Output: Hello
In this example, the variable x
is first assigned the value 5
, and then it is assigned the value "Hello"
. The second assignment replaces the old value 5
with the new value "Hello"
.
However, reusing variable names can make the code harder to understand, especially if the variable is used for different purposes in different parts of the code.
It’s generally better to use descriptive variable names that reflect the purpose of the variable to make the code more readable and maintainable.
Conclusion
Renaming a variable in Python is a common task that can help improve the readability and maintainability of your code. Python provides several ways to rename variables, including using the as
keyword, using tuple unpacking, and using class or instance variables.
When renaming variables, it’s important to follow best practices such as using descriptive variable names, testing the code after renaming, and documenting the changes.
Additionally, code editors and IDEs can make the process of renaming variables faster and more efficient by using features such as Find and Replace.
By following these guidelines, programmers can ensure that their code is easy to understand and maintain, which can save time and effort in the long run.