Have you ever seen that weird arrow in a Python function and thought of the arrow functions in JavaScript.
Well, for me I did, but it turns out that it doesn’t work similarly to the JavaScript arrow function in Python.
The arrow function syntax in JavaScript is a concise way of writing anonymous functions, but in Python, the “->” arrow is used to indicate the return type of a function. This syntax was introduced in Python 3.5 as a way to provide more information about the types of values that functions return.
While the “->” arrow can be used in function annotations to specify the return type, it doesn’t change how the function works or behaves. So, while the syntax may look similar, the arrow function in JavaScript and the “->” arrow in Python serve very different purposes.
So,
What does -> mean in Python?
In Python function definitions, the arrow -> is used for function annotations, specifically to indicate the return type of the function. It allows you to attach metadata to functions describing their parameters and return values. Function annotations can be used to specify the expected type of parameters, and the return type of a function.
Function annotations were introduced in Python 3.x as a way to provide additional information about the function’s arguments and return value.
Function annotations are a feature in Python 3 that allow you to attach metadata to functions that describes their parameters and return values.
Python function annotations
Here is a little bit more information about function annotations in Python:
- Annotations can be used to specify the expected type of a parameter or the return value of a Python function.
- Annotations are defined using the “->” operator, followed by the type or annotation of the return value.
- The annotations are stored in a dictionary named “__annotations__” in the function’s namespace, with the keys being the parameter names and “return” for the return value. The key “return” is used to retrieve the return value annotation for a function.
- Annotations can be any valid Python expression, including simple types like int, str, or bool, or more complex types like classes or functions.
- Annotations can be used to write self-documenting code, and can also be used to validate input parameters and return values, especially when writing tests.
To learn more about function annotations in Python, check out this article: Python Function Annotations
How to annotate return value in Python
So, when you use an arrow in a Python function, you are providing additional information to other developers and future self on the data type that the function should return. Think more of it as a comment, but done at the function definition level.
Here’s an example showing how an arrow -> is used in a Python function that include return data type metadata:
Let’s say you have a function, add_numbers(), that takes in two or more numbers and adds them together.
def add_numbers(a: int, b: int) -> int: return a + b
In this function definition, the function
add_numbers
takes two arguments, both of which are integers, and returns an integer.The type annotations are provided using the
:
syntax, and the return type is indicated using the arrow->
.Function annotations are not enforced by Python itself and are optional, but they can be useful for improving code clarity, catching bugs, and generating documentation. They can also be accessed at runtime using the
__annotations__
attribute of the function object.
Another example here:
Okay, picture this: You’ve got this fancy function called velocity, right? It takes in two arguments, space and time. And then it does some crazy math stuff and spits out the result as velocity.
def velocity(space, time):
return space / time
Now, for those who are not well-versed in the ways of physics, let me break it down the code above for you.
The units of measurement for velocity are in miles per hour (mph), not in units of ‘What the heck did I just calculate?’ or ‘Huh, that’s weird.’
For other programmers who may not understand the units of measurement for velocity, it would be nice to leave them some comment or message about the function. One way to do that is to use the Python arrow function to annotate the function output, which would be the unit of measurement.
With a little bit of code editing, our velocity() function should look like this now:
def velocity(space: int, time: int) -> 'float: in mph':
return space / time
With that, other developers will understand that the return type of the function, velocity()
is a float denoted in miles per hour (mph).
Thus, when writing test code, writing documentation, especially with docstrings, and validating the inputs of the function through logical or conditional statements, programmers will know what to expect from the function, what arguments are required, what arguments are optional, and what data types are acceptable, leading to more efficient and error-free coding.
Besides, write better try … except blocks.
Here would be an updated function that include more meta data, some Docstrings documentation, and effective user input validation.
def velocity(space: int, time: int) -> str:
"""
Calculates the velocity given the space and time.
Args:
- space (int): distance traveled in miles
- time (int): time elapsed in hours
Returns:
- str: velocity in miles per hour (mph), as a string with two decimal places.
"""
try:
v = space / time
return f"{v:.2f} mph"
except ZeroDivisionError:
return "Sorry, cannot divide by zero."
If you call the function, velocity()
while supplying, 20 miles and 2 hours time taken, the function should give you an effective answer, which should be 10.00 mph.
print(velocity(20, 2))
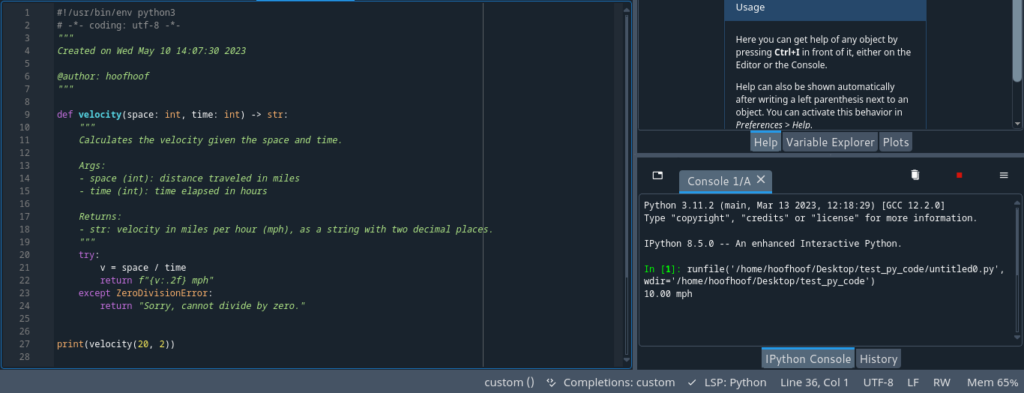
Python function annotations are a powerful tool that can be used to make your code more readable, self-documenting, and easier to understand. By using annotations to specify function parameters and return values, you can make it easier for other developers to work with your code and ensure that your code is robust and maintainable over time.
How to check the return type of a Python function
You can check the return type of a Python function using the __annotations__
attribute. The __annotations__
attribute is a dictionary containing the annotations of the function, including the return type.
Here’s an example:
def add_numbers(a: int, b: int) -> int:
return a + b
return_type = add_numbers.__annotations__['return']
print(return_type) # int
In this example, we defined a function called add_numbers
that takes two arguments, a
and b
, both of type int
. The function is also annotated with the return type of int
.
We then access the __annotations__
attribute of the add_numbers
function to retrieve the annotation for the return type. We extract the return type from the dictionary using the key 'return'
.
Finally, we print the return type, which is 'int'
in this case.
Alternatively, you can check the return type of a Python function using the built-in function type()
on the result of the function call.
For example, if you have a function named add_numbers()
that adds two numbers and returns their sum as an integer, you can check its return type as follows:
def add_numbers(a, b):
return a + b
result = add_numbers(2, 3)
print(type(result)) # Output: <class 'int'>
In this example, the type()
function is used to determine the data type of the result
variable, which stores the return value of the add_numbers()
function.
The output shows that the return value is of type int
, which is what we expect.
If you want to check the return type of a function without actually calling it, you can use the
typing
module’sCallable
type hint.For example, if you have a function
multiply_numbers()
that multiplies two numbers and returns their product as a float, you can useCallable
to specify its return type as follows:from typing import Callable def multiply_numbers(a: int, b: int) -> float: return a * b func: Callable[[int, int], float] = multiply_numbers print(func.__annotations__['return']) # Output: <class 'float'>
In this example, the
Callable
type hint is used to specify the function signature, including the types of its parameters and its return type.The
func
variable is assigned themultiply_numbers()
function, and the__annotations__
attribute is used to access the function annotations and retrieve the return type, which is afloat
.
FAQs
What does -> None do in Python?
-> None in Python function annotations indicates that the function returns nothing, or more specifically, a None
object. This can be useful in cases where the function is called purely for its side effects, such as printing output or modifying a data structure, rather than returning a value for further use in the program.
For example, consider a function that simply prints a message to the console:
def greet(name: str) -> None:
print(f"Hello, {name}!")
In this case, the function takes a name
argument of type str
, but it does not return anything.
The -> None
annotation indicates that the function has no return value. This can help clarify the intended usage of the function and prevent confusion or errors when using it in other parts of the program.
Using -> None
is also a good practice for documenting the behavior of a function, as it indicates that the function does not have any significant return value.
This can be especially important in larger code bases where multiple developers may be working on the same project and need to understand the behavior of different functions.
What does -> str mean in Python function?
-> str in Python function definition is used to indicate that the function is expected to return a string value. This annotation helps other developers to understand the expected data type of the output from the function should be a string.
For example, let’s say we have a function that takes two parameters, ‘name’ and ‘age’, and returns a string that concatenates the two values.
We can define the function using annotations as follows:
def greet(name: str, age: int) -> str:
return f"Hello, my name is {name} and I am {age} years old."
Here, the parameter ‘name’ is expected to be a string and ‘age’ is expected to be an integer. The arrow notation ‘-> str’ indicates that the function is expected to return a string value.
Conclusion
Python function annotations, including the “->” return type annotation and the __annotations__
attribute, are powerful tools that can help make your code more understandable and maintainable. By providing information about the types of function parameters and return values, annotations can help catch potential bugs and improve code functionality.
Additionally, they can be used by external tools, such as linters or IDEs, to provide better code suggestions and autocomplete functionality. Therefore, it’s a good practice to use function annotations in your Python code, and also to check the annotations to ensure the correct data types are being used.